java程序设计扫雷游戏
时间: 2023-12-26 13:28:39 浏览: 133
以下是一个简单的Java程序设计扫雷游戏的示例:
```java
import java.util.Random;
import java.util.Scanner;
public class Minesweeper {
private int rows;
private int cols;
private int numMines;
private char[][] board;
private boolean[][] revealed;
private boolean gameOver;
public Minesweeper(int rows, int cols, int numMines) {
this.rows = rows;
this.cols = cols;
this.numMines = numMines;
this.board = new char[rows][cols];
this.revealed = new boolean[rows][cols];
this.gameOver = false;
initializeBoard();
placeMines();
}
private void initializeBoard() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
board[i][j] = '-';
revealed[i][j] = false;
}
}
}
private void placeMines() {
Random random = new Random();
int count = 0;
while (count < numMines) {
int row = random.nextInt(rows);
int col = random.nextInt(cols);
if (board[row][col] != '*') {
board[row][col] = '*';
count++;
}
}
}
private void revealCell(int row, int col) {
if (row < 0 || row >= rows || col < 0 || col >= cols || revealed[row][col]) {
return;
}
revealed[row][col] = true;
if (board[row][col] == '*') {
gameOver = true;
return;
}
if (board[row][col] == '-') {
int count = countAdjacentMines(row, col);
board[row][col] = (char) (count + '0');
if (count == 0) {
revealCell(row - 1, col - 1);
revealCell(row - 1, col);
revealCell(row - 1, col + 1);
revealCell(row, col - 1);
revealCell(row, col + 1);
revealCell(row + 1, col - 1);
revealCell(row + 1, col);
revealCell(row + 1, col + 1);
}
}
}
private int countAdjacentMines(int row, int col) {
int count = 0;
for (int i = row - 1; i <= row + 1; i++) {
for (int j = col - 1; j <= col + 1; j++) {
if (i >= 0 && i < rows && j >= 0 && j < cols && board[i][j] == '*') {
count++;
}
}
}
return count;
}
private void printBoard() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
if (revealed[i][j]) {
System.out.print(board[i][j] + " ");
} else {
System.out.print("- ");
}
}
System.out.println();
}
}
public void play() {
Scanner scanner = new Scanner(System.in);
while (!gameOver) {
System.out.print("Enter row: ");
int row = scanner.nextInt();
System.out.print("Enter column: ");
int col = scanner.nextInt();
revealCell(row, col);
printBoard();
}
System.out.println("Game over!");
}
public static void main(String[] args) {
Minesweeper game = new Minesweeper(10, 10, 10);
game.play();
}
}
```
阅读全文
相关推荐
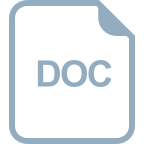
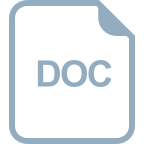
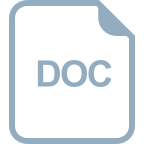
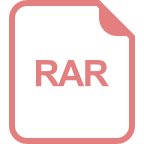
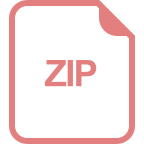
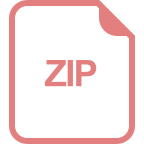
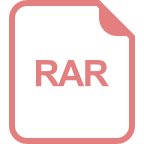
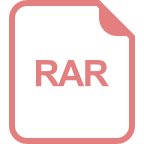
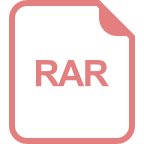
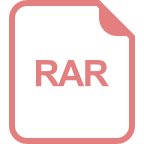
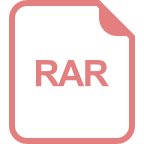
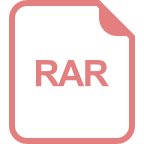
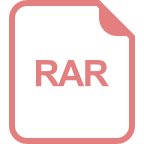
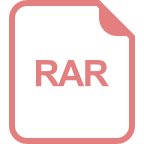
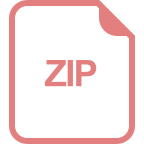
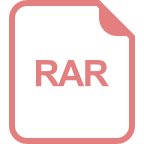
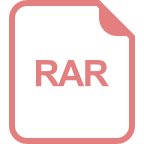