openssl验证证书,c语言代码怎么实现
时间: 2023-06-04 09:02:35 浏览: 446
对于您的问题,我可以提供以下代码实现:
```c
#include <openssl/x509.h>
#include <openssl/pem.h>
int verify_callback(int preverify_ok, X509_STORE_CTX *ctx) {
if (preverify_ok == 0) {
int err = X509_STORE_CTX_get_error(ctx);
printf("Verification failed: %d\n", err);
}
return preverify_ok;
}
int verify_certificate(const char *cert_file_path, const char *ca_cert_file_path) {
int ret = 1;
// Load the certificate from the file
FILE *cert_file = fopen(cert_file_path, "r");
if (cert_file == NULL) {
printf("Failed to open certificate file %s\n", cert_file_path);
return 0;
}
X509 *cert = PEM_read_X509(cert_file, NULL, NULL, NULL);
fclose(cert_file);
// Load the CA certificate from the file
FILE *ca_cert_file = fopen(ca_cert_file_path, "r");
if (ca_cert_file == NULL) {
printf("Failed to open CA certificate file %s\n", ca_cert_file_path);
X509_free(cert);
return 0;
}
X509 *ca_cert = PEM_read_X509(ca_cert_file, NULL, NULL, NULL);
fclose(ca_cert_file);
// Create a certificate store and add the CA certificate
X509_STORE *store = X509_STORE_new();
X509_STORE_add_cert(store, ca_cert);
// Create a verification context and set the callback function
X509_STORE_CTX *ctx = X509_STORE_CTX_new();
X509_STORE_CTX_init(ctx, store, cert, NULL);
X509_STORE_CTX_set_verify_cb(ctx, verify_callback);
// Perform the verification
if (X509_verify_cert(ctx) != 1) {
printf("Certificate verification failed!\n");
ret = 0;
} else {
printf("Certificate verification succeeded!\n");
}
// Clean up
X509_STORE_CTX_free(ctx);
X509_STORE_free(store);
X509_free(cert);
X509_free(ca_cert);
return ret;
}
```
该代码使用 OpenSSL 库实现了对证书的验证,它假定您已经安装了 OpenSSL 库,因此需要包含 `<openssl/x509.h>` 和 `<openssl/pem.h>`。该代码使用 `verify_certificate` 函数,您需要传递要验证的证书文件路径和 CA 证书文件路径。如果验证失败,函数会返回 0,否则会返回 1。注意,该函数在执行之前需要确保证书文件和 CA 证书文件存在且可读。
阅读全文
相关推荐
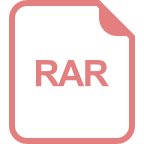
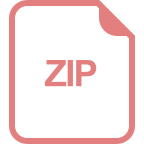


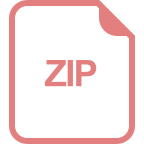
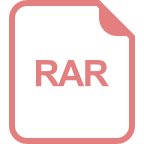
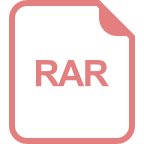
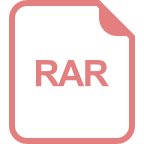
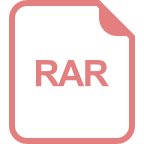
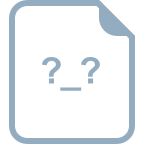
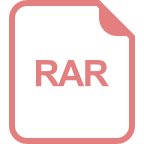
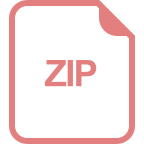
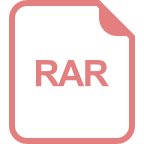
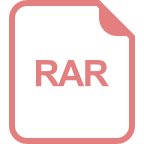
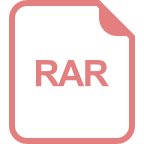
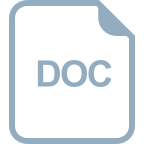
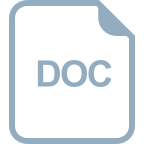
