openssl用于验证签名的C语言代码示例
时间: 2023-07-05 22:04:12 浏览: 155
以下是使用 OpenSSL 库进行签名验证的示例代码。假设您已经有了签名和公钥证书的文件。
```c
#include <stdio.h>
#include <stdlib.h>
#include <openssl/bio.h>
#include <openssl/evp.h>
#include <openssl/pem.h>
int main() {
// 读取签名文件
FILE* f = fopen("signature.bin", "rb");
if (!f) {
perror("Failed to open signature file");
return EXIT_FAILURE;
}
fseek(f, 0, SEEK_END);
long siglen = ftell(f);
rewind(f);
unsigned char* sig = (unsigned char*)malloc(siglen);
fread(sig, 1, siglen, f);
fclose(f);
// 读取公钥文件
BIO* bio = BIO_new_file("public.crt", "r");
if (!bio) {
perror("Failed to open public key file");
return EXIT_FAILURE;
}
X509* cert = PEM_read_bio_X509(bio, NULL, NULL, NULL);
EVP_PKEY* pubkey = X509_get_pubkey(cert);
// 读取要验证的文件
f = fopen("file_to_verify.bin", "rb");
if (!f) {
perror("Failed to open file to verify");
return EXIT_FAILURE;
}
fseek(f, 0, SEEK_END);
long datalen = ftell(f);
rewind(f);
unsigned char* data = (unsigned char*)malloc(datalen);
fread(data, 1, datalen, f);
fclose(f);
// 创建签名上下文并验证签名
EVP_MD_CTX* mdctx = EVP_MD_CTX_new();
EVP_VerifyInit(mdctx, EVP_sha256());
EVP_VerifyUpdate(mdctx, data, datalen);
int result = EVP_VerifyFinal(mdctx, sig, siglen, pubkey);
// 清理资源
EVP_MD_CTX_free(mdctx);
EVP_PKEY_free(pubkey);
X509_free(cert);
BIO_free(bio);
free(sig);
free(data);
// 输出验证结果
if (result == 1) {
printf("Signature verification succeeded!\n");
return EXIT_SUCCESS;
}
else if (result == 0) {
printf("Signature verification failed!\n");
return EXIT_FAILURE;
}
else {
printf("Error occurred during signature verification!\n");
return EXIT_FAILURE;
}
}
```
这是一个简单的示例,您需要根据自己的需求进行修改和扩展。
阅读全文
相关推荐
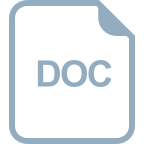
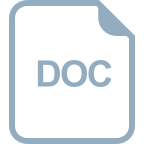
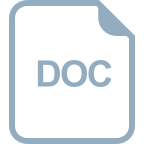


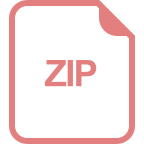
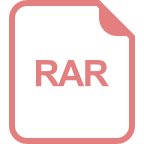
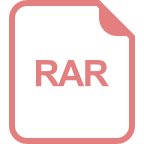
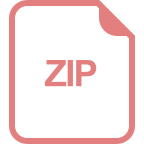









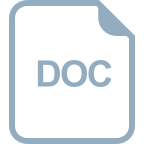