openssl c语言公钥加密
时间: 2024-03-15 19:40:11 浏览: 70
OpenSSL是一个开源的密码学工具库,它提供了一系列的加密、解密、签名和验证等功能。在C语言中使用OpenSSL进行公钥加密可以通过以下步骤实现:
1. 导入OpenSSL库:在C语言中使用OpenSSL库,需要包含相应的头文件,并链接OpenSSL库文件。
```c
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <openssl/err.h>
```
2. 生成RSA密钥对:使用OpenSSL生成RSA密钥对,其中包括公钥和私钥。
```c
RSA *rsa = RSA_generate_key(bits, exp, NULL, NULL);
```
3. 加载公钥和私钥:将生成的公钥和私钥保存到文件中,并加载到程序中。
```c
FILE *pubKeyFile = fopen("public.pem", "rb");
RSA *pubKey = PEM_read_RSA_PUBKEY(pubKeyFile, NULL, NULL, NULL);
fclose(pubKeyFile);
FILE *priKeyFile = fopen("private.pem", "rb");
RSA *priKey = PEM_read_RSAPrivateKey(priKeyFile, NULL, NULL, NULL);
fclose(priKeyFile);
```
4. 加密数据:使用公钥对数据进行加密。
```c
unsigned char encrypted[4098];
int encryptedLen = RSA_public_encrypt(dataLen, data, encrypted, pubKey, padding);
```
5. 解密数据:使用私钥对加密后的数据进行解密。
```c
unsigned char decrypted[4098];
int decryptedLen = RSA_private_decrypt(encryptedLen, encrypted, decrypted, priKey, padding);
```
请注意,以上代码只是一个简单的示例,实际使用时需要进行错误处理和内存释放等操作。
阅读全文
相关推荐
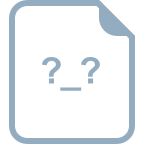
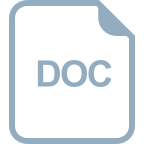
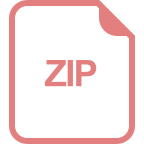
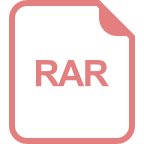
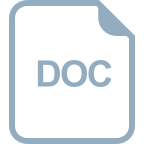








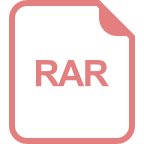
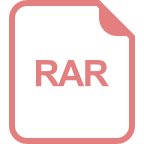
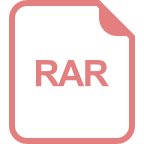
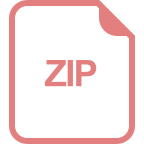