react怎么写openlayers悬浮事件后并添加点击事件
时间: 2024-01-20 07:03:15 浏览: 75
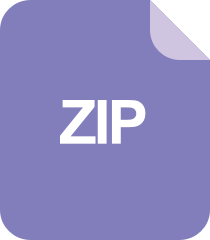
react-open-layers
要在 React 中实现 OpenLayers 的悬浮事件和点击事件,可以按照以下步骤进行操作:
1.在 React 项目中安装 OpenLayers:
```
npm install ol
```
2.在 React 组件中引入 OpenLayers:
```
import 'ol/ol.css';
import Map from 'ol/Map';
import View from 'ol/View';
import TileLayer from 'ol/layer/Tile';
import OSM from 'ol/source/OSM';
import VectorLayer from 'ol/layer/Vector';
import VectorSource from 'ol/source/Vector';
import Feature from 'ol/Feature';
import Point from 'ol/geom/Point';
import { fromLonLat } from 'ol/proj';
import { Style, Fill, Stroke, Circle } from 'ol/style';
```
3.在 React 组件中创建地图和图层:
```
class MapContainer extends React.Component {
constructor(props) {
super(props);
this.mapRef = React.createRef();
}
componentDidMount() {
const map = new Map({
target: this.mapRef.current,
layers: [
new TileLayer({
source: new OSM(),
}),
],
view: new View({
center: fromLonLat([120.1551, 30.2741]),
zoom: 10,
}),
});
const vectorSource = new VectorSource({
features: [
new Feature({
geometry: new Point(fromLonLat([120.1551, 30.2741])),
}),
],
});
const vectorLayer = new VectorLayer({
source: vectorSource,
style: new Style({
image: new Circle({
radius: 6,
fill: new Fill({
color: '#3399CC',
}),
stroke: new Stroke({
color: '#fff',
width: 2,
}),
}),
}),
});
map.addLayer(vectorLayer);
}
render() {
return <div ref={this.mapRef} className="map-container"></div>;
}
}
export default MapContainer;
```
4.在 React 组件中添加悬浮事件和点击事件:
```
class MapContainer extends React.Component {
constructor(props) {
super(props);
this.mapRef = React.createRef();
}
componentDidMount() {
const map = new Map({
target: this.mapRef.current,
layers: [
new TileLayer({
source: new OSM(),
}),
],
view: new View({
center: fromLonLat([120.1551, 30.2741]),
zoom: 10,
}),
});
const vectorSource = new VectorSource({
features: [
new Feature({
geometry: new Point(fromLonLat([120.1551, 30.2741])),
name: 'My Point',
}),
],
});
const vectorLayer = new VectorLayer({
source: vectorSource,
style: new Style({
image: new Circle({
radius: 6,
fill: new Fill({
color: '#3399CC',
}),
stroke: new Stroke({
color: '#fff',
width: 2,
}),
}),
}),
});
map.addLayer(vectorLayer);
// 悬浮事件
const overlay = new Overlay({
element: document.createElement('div'),
});
overlay.setMap(map);
const pointerMoveHandler = function (evt) {
const feature = map.forEachFeatureAtPixel(evt.pixel, function (feature) {
return feature;
});
if (feature) {
const geometry = feature.getGeometry();
const coord = geometry.getCoordinates();
overlay.getElement().innerHTML = feature.get('name');
overlay.setPosition(coord);
} else {
overlay.setPosition(undefined);
}
};
map.on('pointermove', pointerMoveHandler);
// 点击事件
map.on('click', function (evt) {
const feature = map.forEachFeatureAtPixel(evt.pixel, function (feature) {
return feature;
});
if (feature) {
console.log(feature.get('name'));
}
});
}
render() {
return <div ref={this.mapRef} className="map-container"></div>;
}
}
export default MapContainer;
```
在上述代码中,我们首先创建了一个 Overlay 对象并将其添加到地图中,然后在 pointermove 事件处理函数中获取当前鼠标悬浮的要素,并将其名称显示在 Overlay 上。在 click 事件处理函数中,我们可以获取被点击的要素,并打印出其名称。
注意:在 React 中,我们需要使用 `ref` 来获取 DOM 元素的引用。在上述代码中,我们使用 `this.mapRef` 来获取地图容器的引用,并将其传递给 `Map` 构造函数的 `target` 参数中。
阅读全文
相关推荐
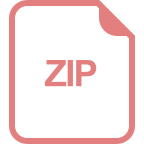
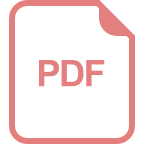


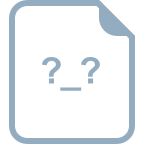
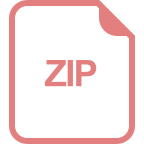
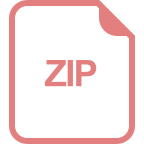
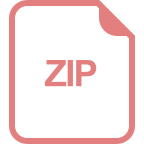
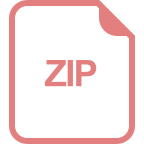




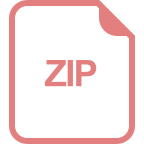
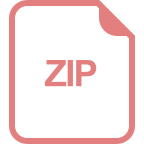
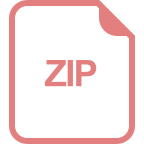
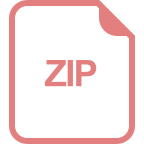
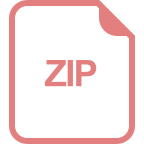