android用sqllite数据库实现诗词的增删改查功能,写一个详细的简单示例,写清文件名
时间: 2023-07-16 22:15:21 浏览: 41
好的,以下是一个简单的 Android 应用程序,使用 SQLite 数据库实现诗词的增删改查功能,包括以下几个文件:
1. `Poem.java`:定义了 `Poem` 类,表示一首诗词。
```java
public class Poem {
private long id;
private String title;
private String author;
private String content;
public Poem() {}
public Poem(String title, String author, String content) {
this.title = title;
this.author = author;
this.content = content;
}
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
}
```
2. `PoemDatabaseHelper.java`:定义了 `PoemDatabaseHelper` 类,继承自 `SQLiteOpenHelper`,用于创建和更新数据库。
```java
public class PoemDatabaseHelper extends SQLiteOpenHelper {
private static final String DATABASE_NAME = "poems.db";
private static final int DATABASE_VERSION = 1;
public PoemDatabaseHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL("CREATE TABLE poems (_id INTEGER PRIMARY KEY AUTOINCREMENT, title TEXT, author TEXT, content TEXT);");
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS poems;");
onCreate(db);
}
}
```
3. `PoemDataSource.java`:定义了 `PoemDataSource` 类,用于操作数据库,包括增删改查功能。
```java
public class PoemDataSource {
private SQLiteDatabase database;
private PoemDatabaseHelper dbHelper;
private String[] allColumns = { "_id", "title", "author", "content" };
public PoemDataSource(Context context) {
dbHelper = new PoemDatabaseHelper(context);
}
public void open() throws SQLException {
database = dbHelper.getWritableDatabase();
}
public void close() {
dbHelper.close();
}
public Poem createPoem(String title, String author, String content) {
ContentValues values = new ContentValues();
values.put("title", title);
values.put("author", author);
values.put("content", content);
long insertId = database.insert("poems", null, values);
Cursor cursor = database.query("poems", allColumns, "_id = " + insertId, null, null, null, null);
cursor.moveToFirst();
Poem newPoem = cursorToPoem(cursor);
cursor.close();
return newPoem;
}
public void deletePoem(Poem poem) {
long id = poem.getId();
database.delete("poems", "_id = " + id, null);
}
public void updatePoem(Poem poem) {
long id = poem.getId();
ContentValues values = new ContentValues();
values.put("title", poem.getTitle());
values.put("author", poem.getAuthor());
values.put("content", poem.getContent());
database.update("poems", values, "_id = " + id, null);
}
public List<Poem> getAllPoems() {
List<Poem> poems = new ArrayList<Poem>();
Cursor cursor = database.query("poems", allColumns, null, null, null, null, null);
cursor.moveToFirst();
while (!cursor.isAfterLast()) {
Poem poem = cursorToPoem(cursor);
poems.add(poem);
cursor.moveToNext();
}
cursor.close();
return poems;
}
private Poem cursorToPoem(Cursor cursor) {
Poem poem = new Poem();
poem.setId(cursor.getLong(0));
poem.setTitle(cursor.getString(1));
poem.setAuthor(cursor.getString(2));
poem.setContent(cursor.getString(3));
return poem;
}
}
```
4. `MainActivity.java`:定义了 `MainActivity` 类,是 Android 应用程序的主界面,用于展示诗词列表、添加诗词等操作。
```java
public class MainActivity extends AppCompatActivity {
private PoemDataSource dataSource;
private List<Poem> poems = new ArrayList<>();
private PoemAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
dataSource = new PoemDataSource(this);
dataSource.open();
ListView listView = findViewById(R.id.list_view);
adapter = new PoemAdapter(this, poems);
listView.setAdapter(adapter);
Button addButton = findViewById(R.id.add_button);
addButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showAddDialog();
}
});
loadPoems();
}
@Override
protected void onResume() {
super.onResume();
dataSource.open();
}
@Override
protected void onPause() {
super.onPause();
dataSource.close();
}
private void loadPoems() {
poems.clear();
poems.addAll(dataSource.getAllPoems());
adapter.notifyDataSetChanged();
}
private void showAddDialog() {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("Add Poem");
View view = getLayoutInflater().inflate(R.layout.dialog_add_poem, null);
final EditText titleEditText = view.findViewById(R.id.title_edit_text);
final EditText authorEditText = view.findViewById(R.id.author_edit_text);
final EditText contentEditText = view.findViewById(R.id.content_edit_text);
builder.setView(view);
builder.setPositiveButton("OK", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
String title = titleEditText.getText().toString();
String author = authorEditText.getText().toString();
String content = contentEditText.getText().toString();
dataSource.createPoem(title, author, content);
loadPoems();
}
});
builder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dialog.dismiss();
}
});
builder.show();
}
private class PoemAdapter extends ArrayAdapter<Poem> {
public PoemAdapter(Context context, List<Poem> poems) {
super(context, 0, poems);
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
if (convertView == null) {
convertView = getLayoutInflater().inflate(R.layout.list_item_poem, parent, false);
}
Poem poem = getItem(position);
TextView titleTextView = convertView.findViewById(R.id.title_text_view);
TextView authorTextView = convertView.findViewById(R.id.author_text_view);
TextView contentTextView = convertView.findViewById(R.id.content_text_view);
titleTextView.setText(poem.getTitle());
authorTextView.setText(poem.getAuthor());
contentTextView.setText(poem.getContent());
return convertView;
}
}
}
```
5. `activity_main.xml`:定义了 `MainActivity` 类的布局。
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<ListView
android:id="@+id/list_view"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:divider="@null"/>
<Button
android:id="@+id/add_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Add"/>
</LinearLayout>
```
6. `dialog_add_poem.xml`:定义了添加诗词对话框的布局。
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<EditText
android:id="@+id/title_edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Title"/>
<EditText
android:id="@+id/author_edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Author"/>
<EditText
android:id="@+id/content_edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Content"/>
</LinearLayout>
```
以上是一个简单的 Android 应用程序,使用 SQLite 数据库实现诗词的增删改查功能,文件名清单如下:
- `Poem.java`
- `PoemDatabaseHelper.java`
- `PoemDataSource.java`
- `MainActivity.java`
- `activity_main.xml`
- `dialog_add_poem.xml`
相关推荐
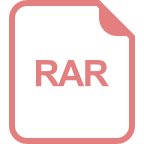
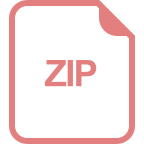
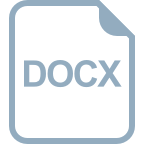














