用android中的sqllite数据库写一个关于古诗的增加、删除,以及按照题目修改和查询功能的笔记本项目,请写一个详细的包括布局文件和java文件的示例
时间: 2024-02-11 13:04:55 浏览: 65
好的,我可以为您提供一个简单的示例。
首先,您需要创建一个包含以下组件的布局文件:
1. 一个EditText用于输入古诗题目
2. 一个EditText用于输入古诗内容
3. 一个添加按钮
4. 一个删除按钮
5. 一个修改按钮
6. 一个查询按钮
7. 一个ListView用于显示所有的古诗列表
接下来,您需要编写一个Java类来处理数据库操作。这个类应该包括以下方法:
1. onCreate()方法用于创建数据库表
2. addPoem()方法用于添加新的古诗
3. deletePoem()方法用于从数据库中删除古诗
4. updatePoem()方法用于修改古诗
5. getAllPoems()方法用于获取所有的古诗列表
6. searchPoem()方法用于根据古诗题目搜索古诗
以下是示例代码:
布局文件 `activity_main.xml`:
```xml
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp">
<EditText
android:id="@+id/edit_title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入古诗题目" />
<EditText
android:id="@+id/edit_content"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入古诗内容" />
<Button
android:id="@+id/btn_add"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="添加" />
<Button
android:id="@+id/btn_delete"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="删除" />
<Button
android:id="@+id/btn_update"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="修改" />
<Button
android:id="@+id/btn_search"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="查询" />
<ListView
android:id="@+id/list_poems"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
```
Java文件 `MainActivity.java`:
```java
public class MainActivity extends AppCompatActivity {
private EditText mTitleEditText;
private EditText mContentEditText;
private Button mAddButton;
private Button mDeleteButton;
private Button mUpdateButton;
private Button mSearchButton;
private ListView mPoemsListView;
private SQLiteDatabase mDatabase;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mTitleEditText = findViewById(R.id.edit_title);
mContentEditText = findViewById(R.id.edit_content);
mAddButton = findViewById(R.id.btn_add);
mDeleteButton = findViewById(R.id.btn_delete);
mUpdateButton = findViewById(R.id.btn_update);
mSearchButton = findViewById(R.id.btn_search);
mPoemsListView = findViewById(R.id.list_poems);
mDatabase = openOrCreateDatabase("poems.db", MODE_PRIVATE, null);
String createTableSql = "CREATE TABLE IF NOT EXISTS poems (id INTEGER PRIMARY KEY AUTOINCREMENT, title TEXT, content TEXT)";
mDatabase.execSQL(createTableSql);
mAddButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String title = mTitleEditText.getText().toString();
String content = mContentEditText.getText().toString();
addPoem(title, content);
mTitleEditText.setText("");
mContentEditText.setText("");
getAllPoems();
}
});
mDeleteButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String title = mTitleEditText.getText().toString();
deletePoem(title);
mTitleEditText.setText("");
getAllPoems();
}
});
mUpdateButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String title = mTitleEditText.getText().toString();
String content = mContentEditText.getText().toString();
updatePoem(title, content);
mTitleEditText.setText("");
mContentEditText.setText("");
getAllPoems();
}
});
mSearchButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String title = mTitleEditText.getText().toString();
searchPoem(title);
}
});
getAllPoems();
}
private void addPoem(String title, String content) {
String insertSql = "INSERT INTO poems (title, content) VALUES (?, ?)";
SQLiteStatement statement = mDatabase.compileStatement(insertSql);
statement.bindString(1, title);
statement.bindString(2, content);
statement.executeInsert();
}
private void deletePoem(String title) {
String deleteSql = "DELETE FROM poems WHERE title = ?";
SQLiteStatement statement = mDatabase.compileStatement(deleteSql);
statement.bindString(1, title);
statement.executeUpdateDelete();
}
private void updatePoem(String title, String content) {
String updateSql = "UPDATE poems SET content = ? WHERE title = ?";
SQLiteStatement statement = mDatabase.compileStatement(updateSql);
statement.bindString(1, content);
statement.bindString(2, title);
statement.executeUpdateDelete();
}
private void getAllPoems() {
String selectSql = "SELECT * FROM poems";
Cursor cursor = mDatabase.rawQuery(selectSql, null);
List<String> poems = new ArrayList<>();
while (cursor.moveToNext()) {
String title = cursor.getString(cursor.getColumnIndex("title"));
String content = cursor.getString(cursor.getColumnIndex("content"));
String poem = title + ": " + content;
poems.add(poem);
}
ArrayAdapter<String> adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, poems);
mPoemsListView.setAdapter(adapter);
}
private void searchPoem(String title) {
String selectSql = "SELECT * FROM poems WHERE title = ?";
Cursor cursor = mDatabase.rawQuery(selectSql, new String[]{title});
List<String> poems = new ArrayList<>();
while (cursor.moveToNext()) {
String content = cursor.getString(cursor.getColumnIndex("content"));
String poem = title + ": " + content;
poems.add(poem);
}
ArrayAdapter<String> adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, poems);
mPoemsListView.setAdapter(adapter);
}
}
```
这个示例还可以进行更多的优化和完善,例如添加数据验证、异常处理等。但这应该足以让您理解如何在Android中使用SQLite数据库来创建一个简单的笔记本应用程序。
阅读全文
相关推荐
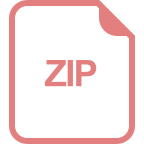





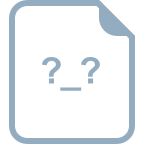
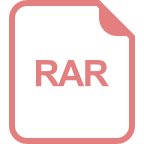
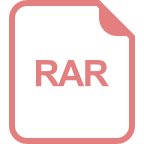
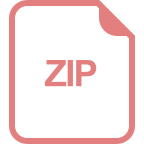
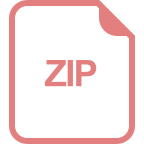





