用android的sqllite数据库写一个古诗词的增、删、查、改,写清楚布局文件、Java文件等相关代码
时间: 2023-07-10 18:03:47 浏览: 98
好的,以下是一个简单的古诗词增删查改的 Android 应用的示例:
布局文件 `activity_main.xml`:
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp">
<EditText
android:id="@+id/edit_title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入标题" />
<EditText
android:id="@+id/edit_author"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入作者" />
<EditText
android:id="@+id/edit_content"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入内容" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center">
<Button
android:id="@+id/btn_add"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="添加" />
<Button
android:id="@+id/btn_update"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="更新" />
<Button
android:id="@+id/btn_delete"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="删除" />
<Button
android:id="@+id/btn_query"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="查询" />
</LinearLayout>
<ListView
android:id="@+id/list"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginTop="16dp" />
</LinearLayout>
```
Java 文件 `MainActivity.java`:
```java
import android.content.ContentValues;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.os.Bundle;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import java.util.ArrayList;
import java.util.List;
public class MainActivity extends AppCompatActivity implements AdapterView.OnItemClickListener, View.OnClickListener {
private EditText editTitle, editAuthor, editContent;
private Button btnAdd, btnUpdate, btnDelete, btnQuery;
private ListView listView;
private ArrayAdapter<String> adapter;
private List<String> dataList = new ArrayList<>();
private DatabaseHelper dbHelper;
private SQLiteDatabase db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
dbHelper = new DatabaseHelper(this, "Poems.db", null, 1);
db = dbHelper.getWritableDatabase();
editTitle = findViewById(R.id.edit_title);
editAuthor = findViewById(R.id.edit_author);
editContent = findViewById(R.id.edit_content);
btnAdd = findViewById(R.id.btn_add);
btnUpdate = findViewById(R.id.btn_update);
btnDelete = findViewById(R.id.btn_delete);
btnQuery = findViewById(R.id.btn_query);
btnAdd.setOnClickListener(this);
btnUpdate.setOnClickListener(this);
btnDelete.setOnClickListener(this);
btnQuery.setOnClickListener(this);
listView = findViewById(R.id.list);
adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, dataList);
listView.setAdapter(adapter);
listView.setOnItemClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.btn_add:
String title = editTitle.getText().toString();
String author = editAuthor.getText().toString();
String content = editContent.getText().toString();
if (!title.isEmpty() && !author.isEmpty() && !content.isEmpty()) {
ContentValues values = new ContentValues();
values.put("title", title);
values.put("author", author);
values.put("content", content);
db.insert("Poems", null, values);
refreshDataList();
Toast.makeText(this, "添加成功!", Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(this, "请输入完整信息!", Toast.LENGTH_SHORT).show();
}
break;
case R.id.btn_update:
title = editTitle.getText().toString();
author = editAuthor.getText().toString();
content = editContent.getText().toString();
if (!title.isEmpty() && !author.isEmpty() && !content.isEmpty()) {
ContentValues values = new ContentValues();
values.put("title", title);
values.put("author", author);
values.put("content", content);
db.update("Poems", values, "title=?", new String[]{title});
refreshDataList();
Toast.makeText(this, "更新成功!", Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(this, "请输入完整信息!", Toast.LENGTH_SHORT).show();
}
break;
case R.id.btn_delete:
title = editTitle.getText().toString();
if (!title.isEmpty()) {
db.delete("Poems", "title=?", new String[]{title});
refreshDataList();
Toast.makeText(this, "删除成功!", Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(this, "请输入标题!", Toast.LENGTH_SHORT).show();
}
break;
case R.id.btn_query:
title = editTitle.getText().toString();
Cursor cursor;
if (!title.isEmpty()) {
cursor = db.query("Poems", null, "title=?", new String[]{title}, null, null, null);
} else {
cursor = db.query("Poems", null, null, null, null, null, null);
}
dataList.clear();
while (cursor.moveToNext()) {
String poemTitle = cursor.getString(cursor.getColumnIndex("title"));
String poemAuthor = cursor.getString(cursor.getColumnIndex("author"));
String poemContent = cursor.getString(cursor.getColumnIndex("content"));
dataList.add(poemTitle + "\n" + poemAuthor + "\n" + poemContent);
}
cursor.close();
adapter.notifyDataSetChanged();
break;
}
}
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
String item = dataList.get(position);
String[] poemInfo = item.split("\n");
editTitle.setText(poemInfo[0]);
editAuthor.setText(poemInfo[1]);
editContent.setText(poemInfo[2]);
}
private void refreshDataList() {
Cursor cursor = db.query("Poems", null, null, null, null, null, null);
dataList.clear();
while (cursor.moveToNext()) {
String title = cursor.getString(cursor.getColumnIndex("title"));
String author = cursor.getString(cursor.getColumnIndex("author"));
String content = cursor.getString(cursor.getColumnIndex("content"));
dataList.add(title + "\n" + author + "\n" + content);
}
cursor.close();
adapter.notifyDataSetChanged();
}
@Override
protected void onDestroy() {
super.onDestroy();
db.close();
}
}
```
Java 文件 `DatabaseHelper.java`:
```java
import android.content.Context;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
public class DatabaseHelper extends SQLiteOpenHelper {
private static final String CREATE_TABLE = "create table Poems ("
+ "id integer primary key autoincrement, "
+ "title text, "
+ "author text, "
+ "content text)";
public DatabaseHelper(Context context, String name, SQLiteDatabase.CursorFactory factory, int version) {
super(context, name, factory, version);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(CREATE_TABLE);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
}
}
```
这个应用中,我们使用了 SQLite 数据库来存储古诗词的信息。具体来说,我们在 `Poems` 表中存储了每首古诗词的标题、作者和内容。在主界面中,我们提供了添加、更新、删除和查询的功能。当用户输入信息并点击添加按钮时,我们通过 `ContentValues` 类将信息插入到数据库中;当用户输入标题并点击查询按钮时,我们通过 `Cursor` 类从数据库中查询出相应的古诗词信息并展示在列表中;当用户点击列表中的某项时,我们将该项对应的古诗词信息填充到输入框中,方便用户进行更新或删除操作。
相关推荐
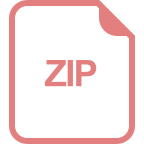
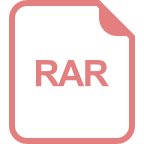














