如何使用C语言编程实现输出当前时间,包括精确到毫秒的显示?
时间: 2024-12-06 15:26:59 浏览: 18
在C语言中,获取当前时间和日期通常依赖于系统库,如`<time.h>`和`<stdio.h>`。要实现精确到毫秒的显示,你需要结合使用`clock()`函数来获取CPU时间戳,以及`strftime()`函数格式化日期和时间。以下是一个简单的示例:
```c
#include <stdio.h>
#include <time.h>
#define MILLIS_PER_SEC (1000)
#define SEC_PER_MINUTE (60)
#define MINUTES_PER_HOUR (60)
// 获取当前时间,并转换为字符串
char* getCurrentTime(long long milliSecs) {
char buffer[25];
time_t rawTime = milliSecs / MILLIS_PER_SEC; // 将毫秒转换为秒
struct tm timeStruct = *localtime(&rawTime); // 转换为tm结构体
strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M:%S", &timeStruct);
buffer[strlen(buffer)-1] = '\0'; // 去除多余的空格
// 添加毫秒部分
int microSecs = (milliSecs % MILLIS_PER_SEC) * 1000;
sprintf(buffer + strlen(buffer), ".%03d", microSecs);
return buffer;
}
int main() {
// 获取当前时间戳(以毫秒为单位)
clock_t startClock = clock();
long long currentTimeMillis = (long long)(CLOCKS_PER_SEC * (double)(startClock / CLOCKS_PER_SEC));
// 输出当前时间
printf("当前时间: %s\n", getCurrentTime(currentTimeMillis));
return 0;
}
```
这个程序首先计算从开始至今的时间戳(以毫秒为单位),然后使用`getCurrentTime`函数将其转换为字符串并格式化。注意,`CLOCKS_PER_SEC`表示每秒的时钟周期数。
阅读全文
相关推荐
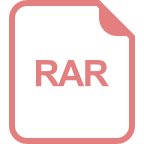
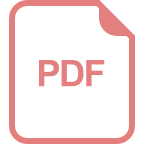
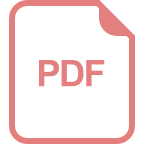

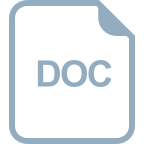
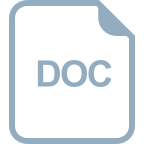
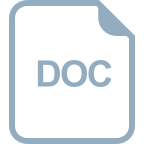
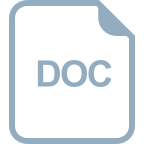
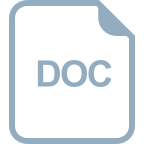
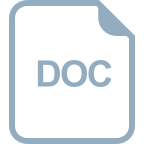
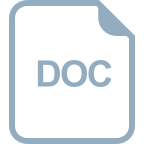
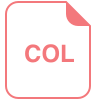



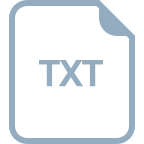
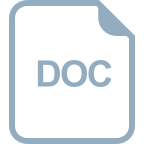
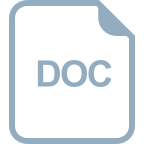
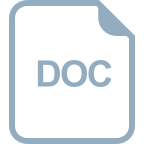