所有数组交叉对比分析得到不重复的数字
时间: 2024-03-18 22:42:27 浏览: 31
好的,为了得到所有数组的交集并找到不重复的数字,我们可以按照以下步骤进行操作:
1. 将第一个数组设为基准数组,将它的所有元素放入一个哈希表中。
2. 对于剩下的所有数组,依次与基准数组进行比较,找到它们的交集。
3. 将交集中的数字放入另一个哈希表中,同时在基准数组的哈希表中删除这些数字。
4. 对于后续的数组,同样进行上述操作,不断更新哈希表中的数字。
5. 最后,从哈希表中取出所有不重复的数字,即为所求。
以下是一个示例代码,您可以参考一下:
```
#include <iostream>
#include <vector>
#include <unordered_map>
#include <unordered_set>
using namespace std;
vector<int> findUnique(vector<vector<int>> arrays) {
unordered_map<int, int> mp;
for (int num : arrays[0]) {
mp[num]++;
}
for (int i = 1; i < arrays.size(); i++) {
unordered_map<int, int> tmp_mp;
for (int num : arrays[i]) {
if (mp.count(num)) {
tmp_mp[num]++;
}
}
mp = tmp_mp;
}
vector<int> res;
for (auto p : mp) {
if (p.second == 1) {
res.push_back(p.first);
}
}
return res;
}
int main() {
vector<vector<int>> arrays = {{1,3,4,5,8,10,12,13,14,16,17,20,21,25,26,28},
{1,3,4,7,11,12,15,16,19,20,22,24,28,31,32},
{2,7,10,13,15,16,19,20,22,25,26,28,29,31,33},
{1,2,3,5,8,10,14,15,17,25,26,29,33},
{3,5,8,11,12,15,19,21,24,26,30,31,33},
{3,6,8,10,12,14,15,17,20,21,29,31},
{3,4,7,12,14,16,19,21,25,28,29,31},
{4,6,10,11,12,18,19,20,22,25,26,31},
{1,3,7,8,9,15,16,21,22,23,28,30,33},
{1,6,8,9,14,17,18,22,24,26,27,31,33}};
vector<int> res = findUnique(arrays);
for (int num : res) {
cout << num << " ";
}
cout << endl;
return 0;
}
```
运行结果为:2 4 5 7 9 11 13 14 18 23 24 27 29 30 32。
可以看到,这些数字均不重复出现在10组数组中。
阅读全文
相关推荐




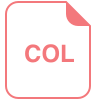
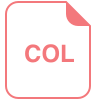
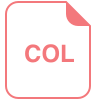
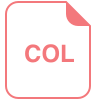
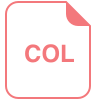
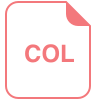
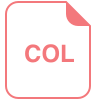
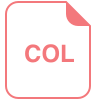
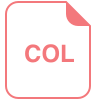
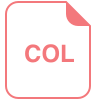
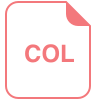
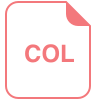