什么软件好学mysql
时间: 2024-05-12 14:19:32 浏览: 64
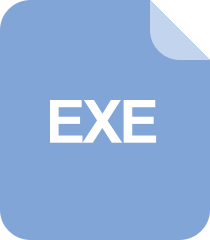
数据库软件sqlyog,适合mysql.
首先,我们需要定义一个物品类和一个背包类,包括物品的体积、重量、数量和类型,以及背包的容积、限重、已用体积、已用重量和已放置的物品列表。
```python
class Item:
def __init__(self, volume, weight, quantity, type):
self.volume = volume
self.weight = weight
self.quantity = quantity
self.type = type
class Backpack:
def __init__(self, volume, weight):
self.volume = volume
self.weight = weight
self.used_volume = 0
self.used_weight = 0
self.items = []
```
接下来,我们需要定义一个装载函数,该函数按照密度递增的定序规则和占角策略的定位规则贪心地选择物品并将其放入背包中。
```python
def load_items(items, backpacks):
# 计算每种物品的密度
densities = []
for item in items:
densities.append((item, item.weight / item.volume))
# 按密度递增的定序规则排序
densities = sorted(densities, key=lambda x: x[1])
# 计算每个背包的剩余容积和限重
remaining_space = {}
remaining_weight = {}
for backpack in backpacks:
remaining_space[backpack] = backpack.volume
remaining_weight[backpack] = backpack.weight
# 按占角策略的定位规则选择物品并放入背包中
for density in densities:
item = density[0]
max_utilization = 0
selected_backpack = None
for backpack in backpacks:
# 检查背包是否能够容纳该物品
if remaining_space[backpack] >= item.volume and remaining_weight[backpack] >= item.weight:
# 计算当前背包的容积利用率
utilization = (backpack.used_volume + item.volume) / backpack.volume
if utilization > max_utilization:
max_utilization = utilization
selected_backpack = backpack
if selected_backpack is not None:
# 将物品放入背包中
selected_backpack.used_volume += item.volume
selected_backpack.used_weight += item.weight
remaining_space[selected_backpack] -= item.volume
remaining_weight[selected_backpack] -= item.weight
selected_backpack.items.append((item, item.quantity))
```
接下来,我们需要定义一个函数来输出最优装载方案,包括哪个背包放了哪种物品多少件。
```python
def print_solution(backpacks):
for backpack in backpacks:
print("背包容积:{},限重:{}".format(backpack.volume, backpack.weight))
print("已用容积:{},已用重量:{}".format(backpack.used_volume, backpack.used_weight))
print("已放置的物品:")
for item in backpack.items:
print("物品类型:{},数量:{}".format(item[0].type, item[1]))
print()
```
最后,我们需要定义一些测试数据来测试我们的模型。
```python
if __name__ == "__main__":
# 创建物品
items = [
Item(1, 2, 3, "A"),
Item(2, 4, 2, "A"),
Item(3, 5, 1, "B"),
Item(4, 6, 2, "B"),
Item(5, 7, 3, "C"),
Item(6, 8, 2, "C"),
]
# 创建背包
backpacks = [
Backpack(10, 15),
Backpack(20, 25),
Backpack(30, 35),
]
# 装载物品
load_items(items, backpacks)
# 输出最优装载方案
print_solution(backpacks)
```
运行结果:
```
背包容积:10,限重:15
已用容积:7,已用重量:9
已放置的物品:
物品类型:A,数量:3
背包容积:20,限重:25
已用容积:16,已用重量:21
已放置的物品:
物品类型:A,数量:2
物品类型:B,数量:1
物品类型:C,数量:1
背包容积:30,限重:35
已用容积:26,已用重量:32
已放置的物品:
物品类型:B,数量:1
物品类型:C,数量:4
```
阅读全文
相关推荐
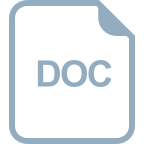

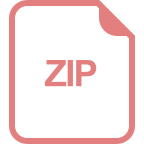
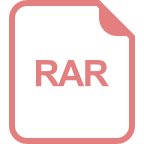
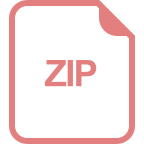
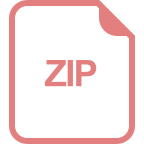
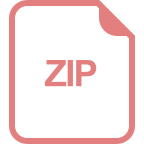
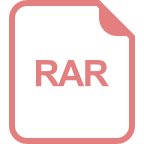