k210 maixpy 多线程运行两个模型的示例
时间: 2024-06-11 09:04:05 浏览: 273
以下是一个示例代码,演示了如何在k210 maixpy平台上使用多线程运行两个模型:
```python
import gc
import sensor
import image
import lcd
import KPU as kpu
import time
from machine import I2C
from fpioa_manager import fm
from board import board_info
from Maix import GPIO
from machine import UART
from machine import Timer
# 初始化I2C总线
fm.register(board_info.PIN10, fm.fpioa.GPIO0)
i2c = I2C(I2C.I2C0, freq=400000, scl=10, sda=11)
devices = i2c.scan()
if devices:
print("I2C addresses found:", [hex(i) for i in devices])
else:
print("No I2C devices found")
# 初始化GPIO口
fm.register(board_info.BUTTON_A, fm.fpioa.GPIO1)
button_a = GPIO(GPIO.GPIO1, GPIO.IN, GPIO.PULL_UP)
# 初始化LCD显示
lcd.init(freq=15000000)
lcd.rotation(2)
# 初始化摄像头
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.set_vflip(1)
sensor.run(1)
# 初始化串口
fm.register(board_info.PIN4, fm.fpioa.UART1_TX, force=True)
fm.register(board_info.PIN5, fm.fpioa.UART1_RX, force=True)
uart1 = UART(UART.UART1, 115200, timeout=1000, read_buf_len=4096)
# 初始化定时器
tim = Timer(Timer.TIMER0, Timer.CHANNEL0, mode=Timer.MODE_PERIODIC, period=1000, unit=Timer.UNIT_MS, callback=lambda timer: print("tick"))
# 加载人脸检测模型
task_detect = kpu.load('/sd/models/multi_face.kmodel')
anchor = (1.889, 2.5245, 2.9465, 3.94056, 3.99987, 5.3658, 5.155437, 6.92275, 6.718375, 9.01025)
kpu.init_yolo2(task_detect, 0.5, 0.3, 5, anchor)
# 加载分类模型
task_classify = kpu.load('/sd/models/mobilenet.kmodel')
# 定义多线程函数
def detect_thread():
while True:
img = sensor.snapshot()
t_start = time.ticks_ms()
# 运行人脸检测模型
faces = kpu.run_yolo2(task_detect, img)
t_stop = time.ticks_ms()
print('Detection time: %dms' % (t_stop - t_start))
# 在LCD上绘制检测结果
lcd.display(img)
lcd.draw_string(0, 0, 'face count: %d' % len(faces), lcd.WHITE, lcd.BLACK)
for i in range(len(faces)):
x, y, w, h = faces[i].rect()
lcd.draw_rectangle(x, y, w, h, lcd.RED, thickness=2)
face_img = img.cut(x, y, w, h)
# 运行分类模型
t_start = time.ticks_ms()
p = kpu.run(task_classify, face_img)
t_stop = time.ticks_ms()
print('Classification time: %dms' % (t_stop - t_start))
# 发送分类结果到串口
uart1.write('face %d: %s\r\n' % (i, p))
def button_thread():
while True:
if button_a.value() == 0:
print("Button A pressed!")
# 开始多线程
import _thread
_thread.start_new_thread(detect_thread, ())
_thread.start_new_thread(button_thread, ())
# 释放资源
gc.collect()
```
该示例代码中,我们通过多线程的方式,同时运行了人脸检测和分类两个模型。其中,`detect_thread`函数用于运行人脸检测模型,并在LCD上绘制检测结果;`button_thread`函数用于监听按钮按下事件。在`detect_thread`函数中,我们首先调用`sensor.snapshot()`方法获取摄像头采集到的图像,然后使用`kpu.run_yolo2`方法运行人脸检测模型,获取检测结果。接着,我们在LCD上绘制检测结果,并针对每个人脸,使用`img.cut`方法获取人脸图像,然后使用`kpu.run`方法运行分类模型,获取分类结果。最后,我们将分类结果发送到串口中。在`button_thread`函数中,我们使用`button_a.value()`方法获取按钮状态,如果按钮被按下,则打印一条提示信息。
需要注意的是,由于k210 maixpy平台内存较小,因此在使用多线程时需要注意内存管理,尤其是在同时运行多个模型时。在示例代码中,我们通过`gc.collect()`方法释放内存。如果需要同时运行更多的模型或任务,可以考虑引入操作系统或使用更高级的开发框架。
阅读全文
相关推荐
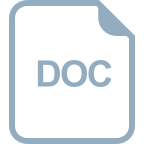
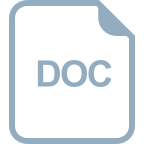
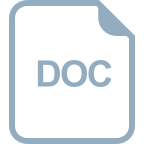

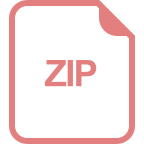


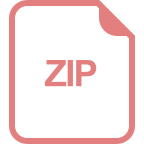
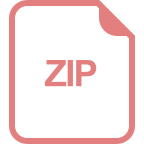
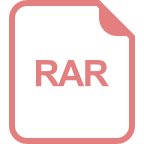
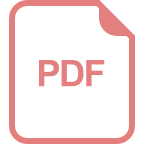
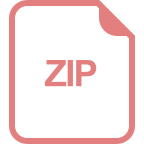






