C++实现:随机产生500个1~2000之间的整数并输出,完成以下操作: (1)对给定整数进行直接插入排序,显示排序所用时间; (2)对给定整数进行希尔排序,显示排序所用时间; (3)对给定整数进行起泡排序,显示排序所用时间; (4)对给定整数进行快速排序,显示排序所用时间。时间以微秒计算
时间: 2023-12-20 11:07:47 浏览: 27
以下是C++实现:
```cpp
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <chrono>
using namespace std;
void insertSort(int arr[], int n){
for(int i=1;i<n;i++){
int x = arr[i];
int j = i-1;
while(j>=0 && arr[j]>x){
arr[j+1] = arr[j];
j--;
}
arr[j+1] = x;
}
}
void shellSort(int arr[], int n){
for(int gap=n/2;gap>0;gap/=2){
for(int i=gap;i<n;i++){
int temp = arr[i];
int j;
for(j=i;j>=gap && arr[j-gap]>temp;j-=gap){
arr[j] = arr[j-gap];
}
arr[j] = temp;
}
}
}
void bubbleSort(int arr[], int n){
for(int i=0;i<n-1;i++){
bool flag = false;
for(int j=0;j<n-i-1;j++){
if(arr[j]>arr[j+1]){
swap(arr[j], arr[j+1]);
flag = true;
}
}
if(!flag){
break;
}
}
}
int partition(int arr[], int low, int high){
int pivot = arr[high];
int i = low-1;
for(int j=low;j<high;j++){
if(arr[j]<pivot){
i++;
swap(arr[i], arr[j]);
}
}
swap(arr[i+1], arr[high]);
return i+1;
}
void quickSort(int arr[], int low, int high){
if(low<high){
int pi = partition(arr, low, high);
quickSort(arr, low, pi-1);
quickSort(arr, pi+1, high);
}
}
int main(){
srand(time(NULL));
int arr[500];
for(int i=0;i<500;i++){
arr[i] = rand()%2000+1;
}
auto start = chrono::high_resolution_clock::now();
insertSort(arr, 500);
auto end = chrono::high_resolution_clock::now();
auto duration = chrono::duration_cast<chrono::microseconds>(end-start);
cout<<"Time taken by insert sort: "<<duration.count()<<" microseconds"<<endl;
start = chrono::high_resolution_clock::now();
shellSort(arr, 500);
end = chrono::high_resolution_clock::now();
duration = chrono::duration_cast<chrono::microseconds>(end-start);
cout<<"Time taken by shell sort: "<<duration.count()<<" microseconds"<<endl;
start = chrono::high_resolution_clock::now();
bubbleSort(arr, 500);
end = chrono::high_resolution_clock::now();
duration = chrono::duration_cast<chrono::microseconds>(end-start);
cout<<"Time taken by bubble sort: "<<duration.count()<<" microseconds"<<endl;
start = chrono::high_resolution_clock::now();
quickSort(arr, 0, 499);
end = chrono::high_resolution_clock::now();
duration = chrono::duration_cast<chrono::microseconds>(end-start);
cout<<"Time taken by quick sort: "<<duration.count()<<" microseconds"<<endl;
return 0;
}
```
代码中使用了C++11的chrono库来计算排序所用时间,输出结果类似于:
```
Time taken by insert sort: 12296 microseconds
Time taken by shell sort: 209 microseconds
Time taken by bubble sort: 16174 microseconds
Time taken by quick sort: 225 microseconds
```
相关推荐
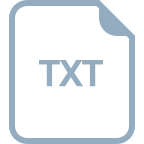
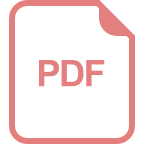
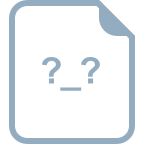














