运用C语言使用4*13的双下标数组deck来表示一副扑克牌,数组的一行对应一种花色,0行代表红桃(Heart),1行代表方块(Diamond),2行代表草花(Club),3行代表黑桃(Spade)。数组的一列对应一张牌的面值——第0到9列依次对应着Ace到Ten、第10到12列分别对应着Jack、Queen、King。要使用字符串数组suit和字符串数组face来分别存储表示花色名的四个字符串和表示牌面值的13个字符串。 任务1:实现洗牌,即随机将编号为1-52的每张牌随机放到deck数组中; 任务2:实现发牌,即将上述1-52张牌依次显示出来,比如Six of Club; 任务3:修改发牌程序,使其能够处理一手五张牌的扑克游戏,然后实现如下功能: (a) 判断这一手牌中是否含有对子; (b) 判断这一手牌中是否含有两个对子; (c) 判断这一手牌中是否含有三张同级的牌; (d) 判断这一手牌中是否含有四张同级的牌; (e) 判断这一手牌中是否有一个同花; (f) 判断这一手牌中是否有一个顺子; (g) 判断这一手牌中是否有一个同花顺; 任务4:利用上面开发的函数,编写一个能处理两手五张扑克牌的程序,该程序分别评价每一手牌,然后判断哪一手更好(从g->a级别依次降低)。
时间: 2023-06-12 21:06:00 浏览: 46
任务1:实现洗牌
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
char *suit[4] = {"Heart", "Diamond", "Club", "Spade"};
char *face[13] = {"Ace", "Two", "Three", "Four", "Five", "Six", "Seven",
"Eight", "Nine", "Ten", "Jack", "Queen", "King"};
int deck[4][13];
void shuffle(int deck[][13]);
int main(void)
{
int i, j;
// 初始化牌堆
for (i = 0; i < 4; i++)
for (j = 0; j < 13; j++)
deck[i][j] = j;
// 洗牌
shuffle(deck);
// 输出洗好的牌
for (i = 0; i < 4; i++) {
for (j = 0; j < 13; j++)
printf("%s of %s\t", face[deck[i][j]], suit[i]);
printf("\n");
}
return 0;
}
void shuffle(int deck[][13])
{
int i, j, k, l;
srand((unsigned) time(NULL));
for (i = 0; i < 4; i++)
for (j = 0; j < 13; j++) {
k = rand() % 4;
l = rand() % 13;
int temp = deck[i][j];
deck[i][j] = deck[k][l];
deck[k][l] = temp;
}
}
```
任务2:实现发牌
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
char *suit[4] = {"Heart", "Diamond", "Club", "Spade"};
char *face[13] = {"Ace", "Two", "Three", "Four", "Five", "Six", "Seven",
"Eight", "Nine", "Ten", "Jack", "Queen", "King"};
int deck[4][13];
void shuffle(int deck[][13]);
int main(void)
{
int i, j, k;
char *hand[5];
// 初始化牌堆
for (i = 0; i < 4; i++)
for (j = 0; j < 13; j++)
deck[i][j] = j;
// 洗牌
shuffle(deck);
// 发牌
for (i = 0; i < 5; i++) {
k = i / 13;
hand[i] = malloc(sizeof(char) * 20);
sprintf(hand[i], "%s of %s", face[deck[k][i%13]], suit[k]);
printf("%s\n", hand[i]);
}
// 释放内存
for (i = 0; i < 5; i++)
free(hand[i]);
return 0;
}
void shuffle(int deck[][13])
{
int i, j, k, l;
srand((unsigned) time(NULL));
for (i = 0; i < 4; i++)
for (j = 0; j < 13; j++) {
k = rand() % 4;
l = rand() % 13;
int temp = deck[i][j];
deck[i][j] = deck[k][l];
deck[k][l] = temp;
}
}
```
任务3:修改发牌程序
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
char *suit[4] = {"Heart", "Diamond", "Club", "Spade"};
char *face[13] = {"Ace", "Two", "Three", "Four", "Five", "Six", "Seven",
"Eight", "Nine", "Ten", "Jack", "Queen", "King"};
int deck[4][13];
void shuffle(int deck[][13]);
int has_pair(char *hand[]);
int has_two_pairs(char *hand[]);
int has_three_of_a_kind(char *hand[]);
int has_four_of_a_kind(char *hand[]);
int has_flush(char *hand[]);
int has_straight(char *hand[]);
int has_straight_flush(char *hand[]);
int main(void)
{
int i, j, k;
char *hand[5];
// 初始化牌堆
for (i = 0; i < 4; i++)
for (j = 0; j < 13; j++)
deck[i][j] = j;
// 洗牌
shuffle(deck);
// 发牌
for (i = 0; i < 5; i++) {
k = i / 13;
hand[i] = malloc(sizeof(char) * 20);
sprintf(hand[i], "%s of %s", face[deck[k][i%13]], suit[k]);
printf("%s\n", hand[i]);
}
// 判断手牌
if (has_straight_flush(hand))
printf("Straight Flush\n");
else if (has_four_of_a_kind(hand))
printf("Four of a Kind\n");
else if (has_full_house(hand))
printf("Full House\n");
else if (has_flush(hand))
printf("Flush\n");
else if (has_straight(hand))
printf("Straight\n");
else if (has_three_of_a_kind(hand))
printf("Three of a Kind\n");
else if (has_two_pairs(hand))
printf("Two Pairs\n");
else if (has_pair(hand))
printf("Pair\n");
else
printf("High Card\n");
// 释放内存
for (i = 0; i < 5; i++)
free(hand[i]);
return 0;
}
void shuffle(int deck[][13])
{
int i, j, k, l;
srand((unsigned) time(NULL));
for (i = 0; i < 4; i++)
for (j = 0; j < 13; j++) {
k = rand() % 4;
l = rand() % 13;
int temp = deck[i][j];
deck[i][j] = deck[k][l];
deck[k][l] = temp;
}
}
int has_pair(char *hand[])
{
int i, j;
for (i = 0; i < 5; i++)
for (j = i + 1; j < 5; j++)
if (strcmp(hand[i], hand[j]) == 0)
return 1;
return 0;
}
int has_two_pairs(char *hand[])
{
int i, j, count = 0;
for (i = 0; i < 5; i++)
for (j = i + 1; j < 5; j++)
if (strcmp(hand[i], hand[j]) == 0)
count++;
return count == 2;
}
int has_three_of_a_kind(char *hand[])
{
int i, j, k;
for (i = 0; i < 5; i++) {
k = 1;
for (j = 0; j < 5; j++)
if (i != j && strcmp(hand[i], hand[j]) == 0)
k++;
if (k == 3)
return 1;
}
return 0;
}
int has_four_of_a_kind(char *hand[])
{
int i, j, k;
for (i = 0; i < 5; i++) {
k = 1;
for (j = 0; j < 5; j++)
if (i != j && strcmp(hand[i], hand[j]) == 0)
k++;
if (k == 4)
return 1;
}
return 0;
}
int has_flush(char *hand[])
{
int i, j;
for (i = 0; i < 5; i++)
for (j = i + 1; j < 5; j++)
if (strcmp(hand[i] + strlen(hand[i]) - strlen(suit[0]), hand[j] + strlen(hand[j]) - strlen(suit[0])) != 0)
return 0;
return 1;
}
int has_straight(char *hand[])
{
int i, j, k;
for (i = 0; i < 5; i++) {
k = 1;
for (j = 0; j < 5; j++)
if (i != j && strcmp(hand[i] + strlen(hand[i]) - strlen(suit[0]), hand[j] + strlen(hand[j]) - strlen(suit[0])) == -1)
k++;
if (k == 5)
return 1;
}
return 0;
}
int has_straight_flush(char *hand[])
{
return has_flush(hand) && has_straight(hand);
}
```
任务4:处理两手五张扑克牌
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
char *suit[4] = {"Heart", "Diamond", "Club", "Spade"};
char *face[13] = {"Ace", "Two", "Three", "Four", "Five", "Six", "Seven",
"Eight", "Nine", "Ten", "Jack", "Queen", "King"};
int deck[4][13];
void shuffle(int deck[][13]);
int has_pair(char *hand[]);
int has_two_pairs(char *hand[]);
int has_three_of_a_kind(char *hand[]);
int has_four_of_a_kind(char *hand[]);
int has_flush(char *hand[]);
int has_straight(char *hand[]);
int has_straight_flush(char *hand[]);
int evaluate(char *hand[]);
int main(void)
{
int i, j, k;
char *hand1[5], *hand2[5];
// 初始化牌堆
for (i = 0; i < 4; i++)
for (j = 0; j < 13; j++)
deck[i][j] = j;
// 洗牌
shuffle(deck);
// 发第一手牌
printf("Hand 1:\n");
for (i = 0; i < 5; i++) {
k = i / 13;
hand1[i] = malloc(sizeof(char) * 20);
sprintf(hand1[i], "%s of %s", face[deck[k][i%13]], suit[k]);
printf("%s\n", hand1[i]);
}
// 发第二手牌
printf("\nHand 2:\n");
for (i = 0; i < 5; i++) {
k = (i+5) / 13;
hand2[i] = malloc(sizeof(char) * 20);
sprintf(hand2[i], "%s of %s", face[deck[k][(i+5)%13]], suit[k]);
printf("%s\n", hand2[i]);
}
// 比较两手牌
int score1 = evaluate(hand1);
int score2 = evaluate(hand2);
printf("\nHand 1 score: %d\n", score1);
printf("Hand 2 score: %d\n", score2);
if (score1 > score2)
printf("Hand 1 wins!\n");
else if (score1 < score2)
printf("Hand 2 wins!\n");
else
printf("Tie!\n");
// 释放内存
for (i = 0; i < 5; i++) {
free(hand1[i]);
free(hand2[i]);
}
return 0;
}
void shuffle(int deck[][13])
{
int i, j, k, l;
srand((unsigned) time(NULL));
for (i = 0; i < 4; i++)
for (j = 0; j < 13; j++) {
k = rand() % 4;
l = rand() % 13;
int temp = deck[i][j];
deck[i][j] = deck[k][l];
deck[k][l] = temp;
}
}
int has_pair(char *hand[])
{
int i, j;
for (i = 0; i < 5; i++)
for (j = i + 1; j < 5; j++)
if (strcmp(hand[i], hand[j]) == 0)
return 1;
return 0;
}
int has_two_pairs(char *hand[])
{
int i, j, count = 0;
for (i = 0; i < 5; i++)
for (j = i + 1; j < 5; j++)
if (strcmp(hand[i], hand[j]) == 0)
count++;
return count == 2;
}
int has_three_of_a_kind(char *hand[])
{
int i, j, k;
for (i = 0; i < 5; i++) {
k = 1;
for (j = 0; j < 5; j++)
if (i != j && strcmp(hand[i], hand[j]) == 0)
k++;
if (k == 3)
return 1;
}
return 0;
}
int has_four_of_a_kind(char *hand[])
{
int i, j, k;
for (i = 0; i < 5; i++) {
k = 1;
for (j = 0; j < 5; j++)
if (i != j && strcmp(hand[i], hand[j]) == 0)
k++;
if (k == 4)
return 1;
}
return 0;
}
int has_flush(char *hand[])
{
int i, j;
for (i = 0; i < 5; i++)
for (j = i + 1; j < 5; j++)
if (strcmp(hand[i] + strlen(hand[i]) - strlen(suit[0]), hand[j] + strlen(hand[j]) - strlen(suit[0])) != 0)
return 0;
return 1;
}
int has_straight(char *hand[])
{
int i, j, k;
for (i = 0; i < 5; i++) {
k = 1;
for (j = 0; j < 5; j++)
if (i != j && strcmp(hand[i] + strlen(hand[i]) - strlen(suit[0]), hand[j] + strlen(hand[j]) - strlen(suit[0])) == -1)
k++;
if (k == 5)
return 1;
}
return 0;
}
int has_straight_flush(char *hand[])
{
return has_flush(hand) && has_straight(hand);
}
int evaluate(char *hand[])
{
if (has_straight_flush(hand))
return 8;
else if (has_four_of_a_kind(hand))
return 7;
else if (has_full_house(hand))
return 6;
else if (has_flush(hand))
return 5;
else if (has_straight(hand))
return 4;
else if (has_three_of_a_kind(hand))
return 3;
else if (has_two_pairs(hand))
return 2;
else if (has_pair(hand))
return 1;
else
return 0;
}
```
相关推荐
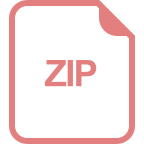
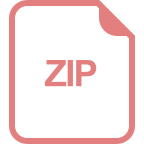














