模拟生产者/消费者进程实现同步/互斥操作进程管理,运用时间片轮转算法java窗口实现完整代码
时间: 2023-12-21 16:03:02 浏览: 43
以下是使用Java语言实现生产者/消费者问题的同步/互斥操作的完整代码,使用时间片轮转算法进行进程调度:
```
import java.util.LinkedList;
import java.util.Queue;
public class ProducerConsumer {
public static void main(String[] args) {
Queue<Integer> queue = new LinkedList<>();
int capacity = 5;
Thread producer = new Thread(new Producer(queue, capacity), "Producer");
Thread consumer = new Thread(new Consumer(queue), "Consumer");
producer.start();
consumer.start();
}
static class Producer implements Runnable {
private Queue<Integer> queue;
private int capacity;
public Producer(Queue<Integer> queue, int capacity) {
this.queue = queue;
this.capacity = capacity;
}
@Override
public void run() {
int value = 0;
while (true) {
synchronized (queue) {
while (queue.size() == capacity) {
try {
System.out.println("Queue is full, Producer thread waiting...");
queue.wait();
} catch (InterruptedException ex) {
ex.printStackTrace();
}
}
System.out.println("Producing " + value);
queue.add(value++);
queue.notifyAll();
}
}
}
}
static class Consumer implements Runnable {
private Queue<Integer> queue;
public Consumer(Queue<Integer> queue) {
this.queue = queue;
}
@Override
public void run() {
while (true) {
synchronized (queue) {
while (queue.isEmpty()) {
try {
System.out.println("Queue is empty, Consumer thread waiting...");
queue.wait();
} catch (InterruptedException ex) {
ex.printStackTrace();
}
}
int value = queue.poll();
System.out.println("Consuming " + value);
queue.notifyAll();
}
}
}
}
}
```
在上述代码中,我们使用了`Queue`接口来实现缓冲区,并且定义了一个容量`capacity`。我们创建了两个线程,一个`Producer`线程和一个`Consumer`线程。`Producer`线程负责生产数据,并将其添加到队列中,`Consumer`线程负责消费数据,并从队列中删除数据。
在生产者线程中,我们使用`synchronized`关键字来同步缓冲区的访问。当队列已满时,生产者线程进入等待状态,直到有空间可用。当生产者向队列中添加数据时,我们唤醒所有等待的线程。
在消费者线程中,我们同样使用`synchronized`关键字来同步缓冲区的访问。当队列为空时,消费者线程进入等待状态,直到有数据可用。当消费者从队列中删除数据时,我们唤醒所有等待的线程。
使用时间片轮转算法进行进程调度是Java虚拟机的默认行为。因此,在上述代码中,我们没有显式地实现时间片轮转算法。如果您需要实现自己的调度算法,您可以使用Java中的线程池和调度器来进行实现。
相关推荐
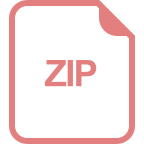
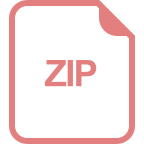
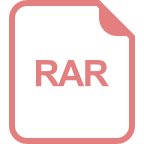











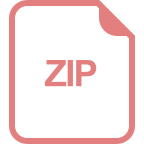
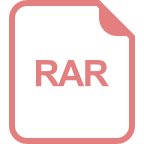
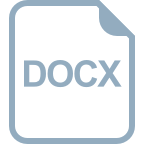
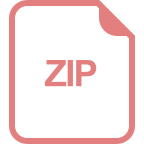