创建两个线程,要求如下: (1)一个线程输出100个1~26,另一个线程输出100个A~Z。 (2)一个线程使用集成Thread 类的写法,另一个线程使用实现Runnable接口的写法。
时间: 2024-02-25 19:54:42 浏览: 104
好的,下面是代码实现:
1. 继承Thread类的写法
```
class PrintNumberThread extends Thread {
@Override
public void run() {
for (int i = 1; i <= 100; i++) {
for (int j = 1; j <= 26; j++) {
System.out.print(j + " ");
}
System.out.println();
}
}
}
class PrintLetterThread extends Thread {
@Override
public void run() {
for (int i = 1; i <= 100; i++) {
for (char j = 'A'; j <= 'Z'; j++) {
System.out.print(j + " ");
}
System.out.println();
}
}
}
public class MultiThreadDemo {
public static void main(String[] args) {
PrintNumberThread numberThread = new PrintNumberThread();
PrintLetterThread letterThread = new PrintLetterThread();
numberThread.start();
letterThread.start();
}
}
```
2. 实现Runnable接口的写法
```
class PrintNumberRunnable implements Runnable {
@Override
public void run() {
for (int i = 1; i <= 100; i++) {
for (int j = 1; j <= 26; j++) {
System.out.print(j + " ");
}
System.out.println();
}
}
}
class PrintLetterRunnable implements Runnable {
@Override
public void run() {
for (int i = 1; i <= 100; i++) {
for (char j = 'A'; j <= 'Z'; j++) {
System.out.print(j + " ");
}
System.out.println();
}
}
}
public class MultiThreadDemo {
public static void main(String[] args) {
PrintNumberRunnable numberRunnable = new PrintNumberRunnable();
PrintLetterRunnable letterRunnable = new PrintLetterRunnable();
Thread numberThread = new Thread(numberRunnable);
Thread letterThread = new Thread(letterRunnable);
numberThread.start();
letterThread.start();
}
}
```
希望这个例子可以帮助你理解Java多线程中的一些概念。
阅读全文
相关推荐
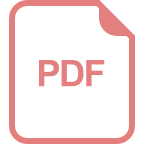
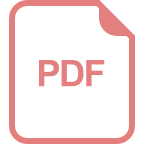
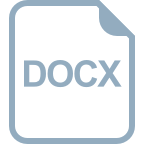
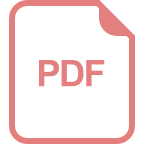
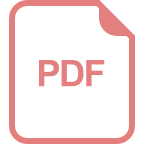
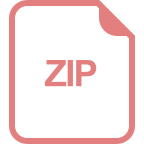
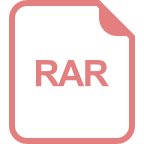
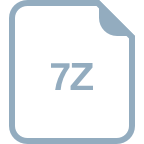
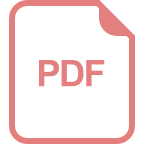
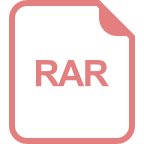
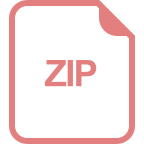
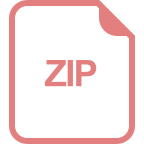
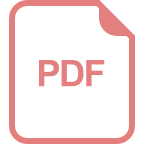
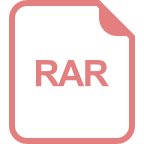
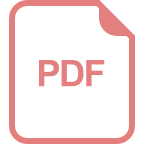
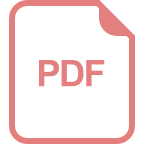
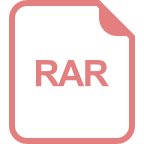
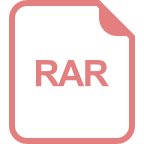