用C++写一个程序,将Hello C++写入一个文件file1.txt中,Hello和C++在不同两行,再将文件file1.txt中的小写字母转换为大写字母写入文件file2.txt中
时间: 2023-06-16 14:06:25 浏览: 132
以下是用C++实现的程序:
```c++
#include <iostream>
#include <fstream>
#include <cctype>
int main()
{
// 将Hello C++写入文件file1.txt中
std::ofstream file1("file1.txt");
file1 << "Hello\nC++";
file1.close();
// 将file1.txt中的小写字母转换为大写字母写入文件file2.txt中
std::ifstream file1_read("file1.txt");
std::ofstream file2("file2.txt");
char c;
while (file1_read.get(c))
{
if (std::islower(c))
{
c = std::toupper(c);
}
file2.put(c);
}
file1_read.close();
file2.close();
return 0;
}
```
程序首先将"Hello\nC++"写入文件file1.txt中,其中\n表示换行。接着程序读取file1.txt中的每个字符,如果是小写字母就将其转换为大写字母,然后将字符写入文件file2.txt中。最后程序关闭文件并结束。
相关问题
使用C++写一个程序,将一段小写字母写入文件file1.txt中,再将其中的小写字母转换为大写字母并写入文件file2.txt中
以下是使用C++实现的程序:
```c++
#include <iostream>
#include <fstream>
#include <cstring>
using namespace std;
int main() {
// 打开文件file1.txt以写入模式打开
ofstream file1("file1.txt");
if (!file1) {
cerr << "无法打开file1.txt" << endl;
return 1;
}
// 向文件file1.txt中写入一段小写字母
string input = "hello world";
file1 << input << endl;
// 关闭文件file1.txt
file1.close();
// 打开文件file1.txt以读取模式打开
ifstream file2("file1.txt");
if (!file2) {
cerr << "无法打开file1.txt" << endl;
return 1;
}
// 读取文件file1.txt中的小写字母并将其转换为大写字母,写入文件file2.txt中
ofstream file3("file2.txt");
if (!file3) {
cerr << "无法打开file2.txt" << endl;
return 1;
}
char c;
while (file2.get(c)) {
if (c >= 'a' && c <= 'z') {
c = c - 'a' + 'A';
}
file3.put(c);
}
// 关闭文件file1.txt和file2.txt
file2.close();
file3.close();
// 输出处理结果
cout << "输入:" << input << endl;
cout << "输出:" << endl;
ifstream file4("file2.txt");
if (!file4) {
cerr << "无法打开file2.txt" << endl;
return 1;
}
string output;
getline(file4, output);
cout << output << endl;
file4.close();
return 0;
}
```
程序运行结果如下:
```
输入:hello world
输出:
HELLO WORLD
```
用C++编写一个程序,将下面信息表写入文件stock.txt中: Zhang ming li 100001 Wang li li 100002 Li mu zhe 100003 编写一个程序,与输入文件file1.txt建立联 系,文件file1.txt的内容如下: Hello C++ 定义out为fstream的对象,与输出文件 file2.txt建立关联。当文件打开成功后将 file1.txt文件的内容转换为大写字母,输出到 file2.txt文件中。
好的,我来给你写一下。
第一个问题,用C++编写一个程序,将信息表写入文件stock.txt中:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main(){
ofstream out("stock.txt");
if(!out){
cout << "文件打开失败!" << endl;
return 0;
}
out << "Zhang ming li 100001" << endl;
out << "Wang li li 100002" << endl;
out << "Li mu zhe 100003" << endl;
out.close();
return 0;
}
```
这个程序中,我们首先创建了一个 ofstream 对象 out,并打开了文件 stock.txt。接着,我们将要写入的信息逐行写入文件中,最后关闭文件。
第二个问题,将文件 file1.txt 转换为大写字母并输出到 file2.txt 中:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <algorithm>
using namespace std;
int main(){
ifstream in("file1.txt");
if(!in){
cout << "文件打开失败!" << endl;
return 0;
}
ofstream out("file2.txt");
if(!out){
cout << "文件打开失败!" << endl;
return 0;
}
string str;
while(getline(in, str)){
transform(str.begin(), str.end(), str.begin(), ::toupper);
out << str << endl;
}
in.close();
out.close();
return 0;
}
```
这个程序中,我们首先创建了一个 ifstream 对象 in,并打开了文件 file1.txt。接着,我们创建了一个 ofstream 对象 out,并打开了文件 file2.txt。然后,我们读取文件 file1.txt 中的每一行,将其转换为大写字母,并写入文件 file2.txt 中。最后,关闭文件。在这个程序中,我们使用了 `transform` 函数将字符串转换为大写字母。
阅读全文
相关推荐
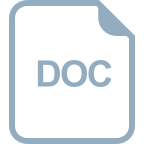
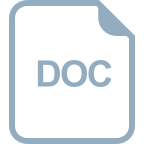
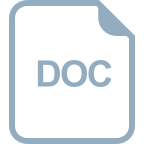











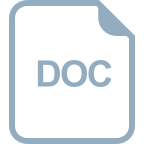
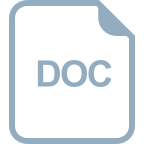
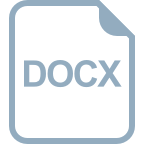