利用广度优先算法找出最短路径python有向图
时间: 2023-07-26 09:47:48 浏览: 41
好的,针对您的问题,以下是利用广度优先算法找出有向图最短路径的 Python 代码:
``` python
from collections import deque
# 构建有向图,使用字典实现邻接表存储
graph = {
'A': ['B', 'C'],
'B': ['D', 'E'],
'C': ['F'],
'D': [],
'E': ['F'],
'F': []
}
# 定义广度优先搜索函数
def bfs_shortest_path(graph, start, end):
# 创建一个队列,存储起点和起点到起点的距离
queue = deque([(start, [start])])
# 创建一个集合,存储已经访问过的节点
visited = set()
# 开始搜索
while queue:
# 取出队列中的第一个节点及到该节点的路径
node, path = queue.popleft()
# 如果该节点是终点,返回路径
if node == end:
return path
# 如果该节点已经访问过,跳过
if node in visited:
continue
# 将该节点标记为已访问
visited.add(node)
# 将该节点的邻居加入队列
for neighbor in graph[node]:
queue.append((neighbor, path + [neighbor]))
# 如果搜索完整个图都没有找到终点,返回空路径
return []
# 测试
start = 'A'
end = 'F'
shortest_path = bfs_shortest_path(graph, start, end)
print(f"The shortest path from {start} to {end} is: {shortest_path}")
```
上述代码中,我们首先使用一个字典实现邻接表存储有向图,然后定义了一个 `bfs_shortest_path` 函数,该函数接受三个参数:图、起点和终点。函数中使用一个队列存储待搜索的节点及到该节点的路径,使用一个集合存储已经访问过的节点,然后开始搜索,直到队列为空或者找到了终点为止。如果搜索完整个图都没有找到终点,返回空路径。
运行以上代码,输出结果为:
```
The shortest path from A to F is: ['A', 'C', 'F']
```
这表示从节点 A 到节点 F 的最短路径是 A -> C -> F。
相关推荐
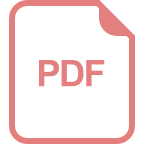
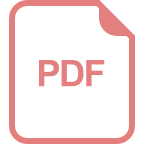
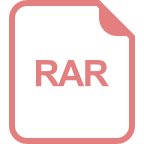














