python单源最短路径问题贪心算法
时间: 2023-11-19 18:53:23 浏览: 77
在Python中,解决单源最短路径问题的贪心算法主要是Dijkstra算法。Dijkstra算法是一种广度优先搜索算法,用于解决有向图或无向图的单源最短路径问题,且不能出现权值为负的情况。该算法通过不断选择距离起点最近的顶点,来逐渐扩大最短路径权值,直到覆盖图中所有顶点。其应用根本在于最短路径的最优子结构性质。Dijkstra算法步骤与Floyd算法类似,更新规则也是dist[j]=min(dist[j],dist[i]+matrix[i][j])。
具体实现时,可以使用Python中的heapq模块来实现优先队列,以提高算法效率。以下是一个简单的Python实现Dijkstra算法的例子:
```
import heapq
def dijkstra(graph, start):
# 初始化距离字典
dist = {node: float('inf') for node in graph}
dist[start] = 0
# 初始化堆
heap = [(0, start)]
while heap:
# 弹出堆中距离最小的节点
(distance, node) = heapq.heappop(heap)
# 如果当前节点已经被访问过,则跳过
if distance > dist[node]:
continue
# 遍历当前节点的邻居节点
for neighbor, weight in graph[node].items():
# 计算到邻居节点的距离
new_distance = dist[node] + weight
# 如果新的距离比原来的距离小,则更新距离字典和堆
if new_distance < dist[neighbor]:
dist[neighbor] = new_distance
heapq.heappush(heap, (new_distance, neighbor))
return dist
```
相关推荐
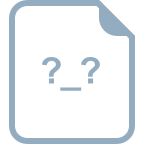














