img = imread('org_1.png'); gray_image = rgb2gray(img); edge_image = edge(gray_image, 'Canny', 0.1); filled_image = imfill(edge_image, 'holes'); cc = bwconncomp(filled_image); stats = regionprops(cc, 'Area', 'BoundingBox'); areas = [stats.Area]; [~, idx] = sort(areas, 'descend'); bounding_box = stats(idx(1)).BoundingBox; % 识别色块颜色 result = cell(4,4); for i = 1 : 4 for j = 1 : 4 % 计算每个小方块的位置 x1 = bounding_box(1) + (j - 1) * bounding_box(3) / 4; x2 = bounding_box(1)+ j * bounding_box(3) / 4; y1 = bounding_box(2) + (i - 1) * bounding_box(4) / 4; y2 = bounding_box(2) + i * bounding_box(4) / 4; % 从原图中截取当前块 block = img(y1:y2, x1:x2, :); % 颜色识别 [r, g, b] = getColor(block); color_block = ''; if r > g && r > b % 红色 color_block = 'r'; elseif g > r && g > b % 绿色 color_block = 'g'; elseif b > r && b > g % 蓝色 color_block = 'b'; end if r > 128 && g > 128 % 黄色 color_block = 'y'; end % 将识别结果存入结果数组 result{i, j} = color_block; end end fprintf('result=\n'); % 输出结果 for i = 1 : 4 for j = 1 : 4 fprintf('%s\t', result{i, j}); end fprintf('\n'); end % 颜色识别函数 function [r, g, b] = getColor(block) % 提取每个通道中像素的平均值 r = mean(mean(block(:, :, 1))); g = mean(mean(block(:, :, 2))); b = mean(mean(block(:, :, 3))); end
时间: 2024-03-11 17:44:43 浏览: 18
这段代码实现了一种基于颜色识别的 Rubik's Cube(魔方)解法,具体流程如下:
1. 读入原始图片 `org_1.png`,将其转换成灰度图 `gray_image`,再利用 Canny 边缘检测算法得到边缘图 `edge_image`。
2. 对边缘图执行洞填充操作 `imfill`,得到填充后的图像 `filled_image`。
3. 对填充后的图像进行连通区域分析 `bwconncomp`,得到各个连通区域的信息 `stats`。
4. 对各个连通区域按面积从大到小排序,取面积最大的连通区域,即为 Rubik's Cube 所在的区域。
5. 将 Rubik's Cube 区域分割成 16 个小块,对每个小块进行颜色识别。
6. 对每个小块进行颜色识别,识别方法为:提取每个通道中像素的平均值,判断红、绿、蓝、黄四种颜色的像素值,颜色识别结果存入结果数组 `result`。
7. 输出最终结果 `result`。
颜色识别函数 `getColor` 实现了从 RGB 图像中提取颜色信息的功能,具体实现方法为:提取每个通道中像素的平均值,得到红、绿、蓝三个通道的平均值,利用这些平均值判断颜色类型。其中,如果红色通道和绿色通道的平均值都大于 128,则判定为黄色。
需要注意的是,这段代码对 Rubik's Cube 的颜色识别方法较为简单,只针对四种基本颜色进行识别,对于其他颜色则无法识别。如果需要更精确的颜色识别,可以采用其他方法,如 HSV 颜色空间分析等。
相关问题
改错clc,clear;close all %% step1读取并显示右图像: I = imread('p1.png'); %% step2图像预处理: imhist(I)%可以显示I图像的直方图 subplot(1,5,1),imshow(I);%显示原图像 subplot(1,5,2),imhist(I)%显示其直方图 I=rgb2gray(I); imshow(I) % 滤波 filtered_img = medfilt2(I); % 中值滤波器 % 或者使用高斯滤波器 % filtered_img = imgaussfilt(img); % 直方图均衡化 enhanced_img = histeq(filtered_img); % 显示处理后的图像 subplot(1,5,3); imshow(filtered_img); title('Filtered Image'); subplot(1,5,4); imshow(enhanced_img); title('Enhanced Image'); %% step3边界检测 BW3 = edge(I, 'canny');%使用canny方法检测边缘 subplot(1,5,5); imshow(BW3) %% step4边界分析: % 提取边界 boundaries = bwboundaries(I); % 显示原始图像和提取的边界 figure; subplot(1,2,1); imshow(I); title('Binary Image'); subplot(1,2,2); imshow(boundaries); hold on; for k = 1:length(boundaries) boundary = boundaries{k}; plot(boundary(:,2), boundary(:,1), 'r', 'LineWidth', 2); end title('Boundaries'); hold off;
这是一个已经修改过的代码,包括添加了 `clc`、`clear` 和 `close all` 来清除命令窗口和关闭所有图像窗口。
```matlab
clc;
clear;
close all;
%% step1读取并显示右图像:
I = imread('p1.png');
%% step2图像预处理:
imhist(I); %可以显示I图像的直方图
subplot(1,5,1),imshow(I); %显示原图像
subplot(1,5,2),imhist(I); %显示其直方图
I = rgb2gray(I);
imshow(I);
% 滤波
filtered_img = medfilt2(I); % 中值滤波器
% 或者使用高斯滤波器
% filtered_img = imgaussfilt(I);
% 直方图均衡化
enhanced_img = histeq(filtered_img);
% 显示处理后的图像
subplot(1,5,3);
imshow(filtered_img);
title('Filtered Image');
subplot(1,5,4);
imshow(enhanced_img);
title('Enhanced Image');
%% step3边界检测
BW3 = edge(I, 'canny'); %使用canny方法检测边缘
subplot(1,5,5);
imshow(BW3);
%% step4边界分析:
% 提取边界
boundaries = bwboundaries(BW3);
% 显示原始图像和提取的边界
figure;
subplot(1,2,1);
imshow(I);
title('Binary Image');
subplot(1,2,2);
imshow(I);
hold on;
for k = 1:length(boundaries)
boundary = boundaries{k};
plot(boundary(:,2), boundary(:,1), 'r', 'LineWidth', 2);
end
title('Boundaries');
hold off;
```
这个代码会按照以下步骤进行图像预处理、边界检测和边界分析:
1. 读取并显示右图像。
2. 显示原图像和其直方图,然后将图像转换为灰度图像。
3. 应用中值滤波或高斯滤波对图像进行平滑处理,并进行直方图均衡化。显示处理后的图像。
4. 使用Canny方法检测图像的边缘,并显示结果。
5. 提取边界,并在原始图像上显示提取的边界。
请注意,你需要将图像文件 `'p1.png'` 替换为你实际使用的图像文件,并根据需要调整代码中的其他参数和参数值。
matlab图像边缘检测
在MATLAB中,可以使用不同的边缘检测算法来检测图像中的边缘。以下是使用Sobel算子和Canny算子进行边缘检测的示例代码:
使用Sobel算子进行边缘检测:
```matlab
% 读取图像
img = imread('lena.png');
% 转换为灰度图像
gray_img = rgb2gray(img);
% 使用Sobel算子进行边缘检测
sobel_img = edge(gray_img, 'sobel');
% 显示结果
figure;
subplot(1,2,1), imshow(gray_img), title('Original Image');
subplot(1,2,2), imshow(sobel_img), title('Sobel Edge Detection');
```
使用Canny算子进行边缘检测:
```matlab
% 读取图像
img = imread('lena.png');
% 转换为灰度图像
gray_img = rgb2gray(img);
% 使用Canny算子进行边缘检测
canny_img = edge(gray_img, 'canny');
% 显示结果
figure;
subplot(1,2,1), imshow(gray_img), title('Original Image');
subplot(1,2,2), imshow(canny_img), title('Canny Edge Detection');
```
以上代码中,`edge`函数用于进行边缘检测,第一个参数为灰度图像,第二个参数为所使用的算子类型。可以通过调整算子类型和参数来获取更好的边缘检测效果。
相关推荐
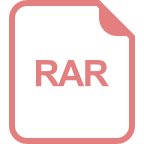
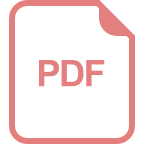
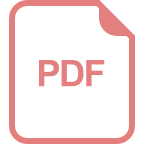












