刚刚的例子中不使用reinterpret_cast转换会有问题吗
时间: 2023-08-16 18:02:35 浏览: 54
在上述示例中,我们没有使用 `reinterpret_cast` 进行类型转换,而是直接使用了 `QDataStream` 的 `<<` 运算符来写入结构体的成员变量。
Qt 的 `QDataStream` 类会根据不同的数据类型自动进行适当的序列化和反序列化操作,以确保数据的正确性和一致性。因此,在常见的数据类型(如整数、浮点数、字符串等)上,你不需要显式使用 `reinterpret_cast` 进行类型转换。
然而,需要注意的是,在某些情况下,如果你想要将自定义的结构体或类对象直接写入文件,并在读取时进行还原,可能需要实现相关的序列化和反序列化操作。这时可能需要使用 `reinterpret_cast` 或其他类型转换方式来处理自定义类型的内存。
总结起来,对于常见的数据类型,Qt 的数据流类会自动处理类型转换,而不需要显式使用 `reinterpret_cast`。但对于复杂的自定义类型,可能需要进行额外的序列化和反序列化操作,并根据需要使用 `reinterpret_cast` 或其他类型转换方式来处理内存。
希望这能解答你的疑问!如果还有其他问题,请随时提问。
相关问题
QT中reinterpret_cast的使用
在QT中,reinterpret_cast是一种类型转换操作符,它用于将一个指针或引用转换为不同类型的指针或引用。它可以用于转换任意类型的指针,甚至是不相关的类型。
使用reinterpret_cast时需要注意以下几点:
1. 由于reinterpret_cast执行的是一种低级别的转换,它没有进行类型检查,所以需要确保转换是合法的。转换的两个类型之间应具有相同的大小和二进制表示,否则行为是未定义的。
2. reinterpret_cast通常用于处理底层的指针或引用,例如将一个指针转换为整数类型以进行一些位操作。但是,在正常情况下,应该优先考虑使用更安全的类型转换操作符,如static_cast。
下面是一个使用reinterpret_cast的例子:
```cpp
int main() {
int value = 10;
int* ptr = &value;
// 将int指针转换为void指针
void* voidPtr = reinterpret_cast<void*>(ptr);
// 将void指针转换回int指针
int* intPtr = reinterpret_cast<int*>(voidPtr);
return 0;
}
```
在上面的例子中,我们首先将int指针ptr转换为void指针voidPtr,然后再将void指针voidPtr转回int指针intPtr。
C++ 中的 reinterpret_cast
C++ 中的 `reinterpret_cast` 是一种类型转换运算符,用于将一个指针或引用转换为不同类型的指针或引用。它可以将一个指针或引用类型转换为任何其他类型的指针或引用类型,包括不相关的类型。这种转换通常是非类型安全的,因为它可以将一个指针或引用转换为不兼容的类型,导致未定义的行为。
`reinterpret_cast` 的语法如下:
```cpp
reinterpret_cast<type-id>(expression)
```
其中,`type-id` 是要转换成的指针或引用类型,`expression` 是要转换的指针或引用。
下面是一个使用 `reinterpret_cast` 进行类型转换的例子:
```cpp
int i = 10;
double* pd = reinterpret_cast<double*>(&i);
```
这个例子中,我们将一个 `int*` 类型的指针转换为 `double*` 类型的指针,虽然这种转换是非法的,但是编译器不会报错。这是因为 `reinterpret_cast` 不会检查转换的合法性,而是将指针或引用的位模式重新解释为另一种类型的位模式。
需要注意的是,在实际应用中,应该避免使用 `reinterpret_cast` 进行类型转换,除非确实需要这种非类型安全的转换。如果需要进行类型转换,应该使用 `dynamic_cast`、`static_cast` 或 `const_cast` 等类型安全的转换方式。
相关推荐
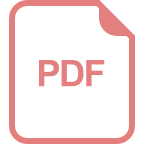
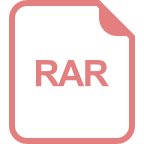
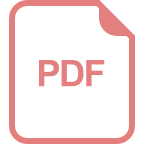












