struct time { int year; /*年* / int month; / *月* / int day; / *日* / } ; 第7章 结构体与共用体 1 5 1 下载 byte[0] data.year byte[1] byte[2] data.month byte[3] byte[4] data.day byte[5] 共 用 体 类 型 1000 1001 1002 1003 1004 1005 存储器 union dig { struct time data; /*嵌套的结构体类型* / char byte[6]; } ; m a i n ( ) { union dig unit; int i; printf("enter year:\n"); s c a n f ( " % d " , & u n i t . d a t a . y e a r ) ; / *输入年* / printf("enter month:\n"); s c a n f ( " % d " , & u n i t . d a t a . m o n t h ) ; / *输入月* / printf("enter day:\n"); s c a n f ( " % d " , & u n i t . d a t a . d a y ) ; / *输入日* / p r i n t f ( " y e a r = % d month=%d day=%d\n", unit.data.year,unit. data. month, unit. d a t a . d a y ) ; / *打印输出* / f o r ( i = 0 ; i < 6 ; i + + ) p r i n t f ( " % d , " , u n i t . b y t e [ i ] ) ; / *按字节以十进制输出* / p r i n t f ( " \ n " ) ; }
时间: 2024-03-19 19:42:25 浏览: 30
这段代码是一个使用结构体和共用体的示例,定义了一个名为time的结构体,包含年月日三个成员变量,然后定义了一个名为dig的共用体,其中包含一个嵌套的time结构体和一个char类型的byte数组,共用体的作用是让同一段内存可以被不同的类型访问。
在主函数中,首先通过scanf函数输入年月日三个变量,然后分别使用结构体和共用体的成员访问方式输出这三个变量的值,最后使用for循环和数组下标访问方式以字节形式输出共用体的内存内容。
需要注意的是,共用体内的内存是按照字节顺序存储的,所以在输出时需要按照字节顺序逐个输出。另外,共用体的大小是其内部最大成员的大小,本例中共用体的大小是6个字节。
相关问题
struct time { int year; /*年* / int month; / *月* / int day; / *日* / } ; 第7章 结构体与共用体 1 5 1 下载 byte[0] data.year byte[1] byte[2] data.month byte[3] byte[4] data.day byte[5] 共 用 体 类 型 1000 1001 1002 1003 1004 1005 存储器 union dig { struct time data; /*嵌套的结构体类型* / char byte[6]; } ; m a i n ( ) { union dig unit; int i; printf("enter year:\n"); s c a n f ( " % d " , & u n i t . d a t a . y e a r ) ; / *输入年* / printf("enter month:\n"); s c a n f ( " % d " , & u n i t . d a t a . m o n t h ) ; / *输入月* / printf("enter day:\n"); s c a n f ( " % d " , & u n i t . d a t a . d a y ) ; / *输入日* / p r i n t f ( " y e a r = % d month=%d day=%d\n", unit.data.year,unit. data. month, unit. d a t a . d a y ) ; / *打印输出* / f o r ( i = 0 ; i < 6 ; i + + ) p r i n t f ( " % d , " , u n i t . b y t e [ i ] ) ; / *按字节以十进制输出* / p r i n t f ( " \ n " ) ; }改成可运行代码
这段代码已经是可运行的代码了,只需要将其复制到C语言的编译器中进行编译和运行即可。需要注意的是,代码中可能存在中文符号,需要将其转换为英文符号才能通过编译器的语法检查。同时,代码中的注释也需要改为C语言的注释格式,即使用双斜线“//”作为注释符号。下面是修改后的代码:
```
#include <stdio.h>
#include <stdlib.h>
struct time {
int year; /*年*/
int month; /*月*/
int day; /*日*/
};
union dig {
struct time data; /*嵌套的结构体类型*/
char byte[6];
};
int main() {
union dig unit;
int i;
printf("enter year:\n");
scanf("%d", &unit.data.year); /*输入年*/
printf("enter month:\n");
scanf("%d", &unit.data.month); /*输入月*/
printf("enter day:\n");
scanf("%d", &unit.data.day); /*输入日*/
printf("year = %d month = %d day = %d\n", unit.data.year, unit.data.month, unit.data.day); /*打印输出*/
for (i = 0; i < 6; i++) {
printf("%d, ", unit.byte[i]); /*按字节以十进制输出*/
}
printf("\n");
return 0;
}
```
C语言/* 函数sum_day:计算日期 */
以下是一个计算日期的示例代码:
```c
#include <stdio.h>
struct Date {
int year;
int month;
int day;
};
int isLeapYear(int year); // 判断是否为闰年
int getDaysInMonth(int year, int month); // 获取该月的天数
int sum_day(struct Date date); // 计算日期
int main() {
struct Date date = {2021, 8, 18};
int days = sum_day(date);
printf("%d年%d月%d日是该年的第%d天\n", date.year, date.month, date.day, days);
return 0;
}
int isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int getDaysInMonth(int year, int month) {
int daysInMonth[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; // 每个月的天数
if (month == 2 && isLeapYear(year)) {
return 29;
} else {
return daysInMonth[month - 1];
}
}
int sum_day(struct Date date) {
int days = 0;
for (int i = 1; i < date.month; i++) {
days += getDaysInMonth(date.year, i);
}
days += date.day;
return days;
}
```
解释一下,首先定义了一个 `Date` 结构体用来存储日期信息。然后定义了两个函数,一个是判断闰年的函数 `isLeapYear`,另一个是获取该月的天数的函数 `getDaysInMonth`。在 `getDaysInMonth` 函数中,先定义了数组 `daysInMonth` 存储每个月的天数,然后根据是否为闰年来修改二月的天数。最后根据月份返回天数。在 `sum_day` 函数中,使用循环计算出累加到该月的天数,最后再加上当月的天数。
相关推荐
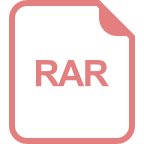
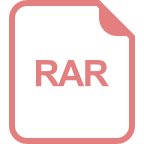
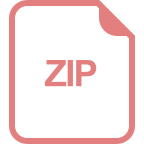












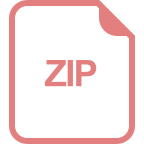
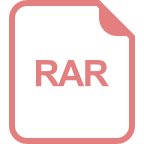
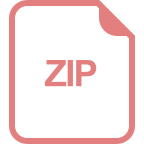