编写一个使用requests+BeautifulSoup的python爬虫爬取页面的模板
时间: 2023-06-12 17:04:00 浏览: 240
以下是一个使用requests和BeautifulSoup库的Python爬虫爬取页面的模板代码:
```python
import requests
from bs4 import BeautifulSoup
# 设置请求头,模拟浏览器访问
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 发起请求
response = requests.get(url, headers=headers)
# 解析网页内容
soup = BeautifulSoup(response.text, 'html.parser')
# 找到需要的信息
result = soup.find('div', class_='result')
# 输出结果
print(result.text)
```
在上述代码中,首先我们定义了一个请求头,用于模拟浏览器访问。然后使用requests库发送请求,获取网页的源代码。接着,使用BeautifulSoup库解析网页的HTML内容,找到需要的信息。最后,将结果打印输出。如果要获取多个结果,可以使用soup.find_all()方法。
相关问题
帮我写一段代码,用python爬虫爬取京东https://mall.jd.com/view_search-1736571-25176241-99-1-20-1.html,返回销量前二百的商品的链接名字和价格
为了编写一个Python爬虫来抓取京东商品页面的数据,我们将使用requests库来发送HTTP请求,并结合BeautifulSoup解析HTML内容。请注意,京东通常会实施反爬虫策略,频繁访问可能会导致IP受限。在实际操作中,你需要遵守网站的robots.txt规则并确保你的爬虫行为符合法规。
这是一个简单的示例,展示如何获取销量前200的商品链接名称和价格,但实际上京东的商品详情页结构可能会有所不同,这只是一个基础的模板:
```python
import requests
from bs4 import BeautifulSoup
# 目标URL
url = "https://mall.jd.com/view_search-1-20-1.html"
def get_html(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
response = requests.get(url, headers=headers)
return response.text if response.status_code == 200 else None
def parse_html(html):
soup = BeautifulSoup(html, 'lxml')
# 这里需要找到商品列表的具体元素,通常是通过CSS选择器或XPath表达式,这取决于京东页面的实际结构
products = soup.find_all('.product-item')[:200] # 取前200个商品
result = []
for product in products:
name = product.find('div', class_='name').text.strip() # 商品名称
price = product.find('span', class_='price').text.strip() # 商品价格
result.append((name, price))
return result
html_content = get_html(url)
if html_content:
data = parse_html(html_content)
print("商品链接和价格:")
for i, (link_name, price) in enumerate(data, start=1):
print(f"{i}. 链接:{link_name}, 价格:{price}")
else:
print("无法获取到HTML内容,检查网络连接或目标URL。")
python爬虫模板
以下是一个简单的Python爬虫完整代码模板,用于演示如何使用requests库和BeautifulSoup库爬取网页内容:
```python
import requests
from bs4 import BeautifulSoup
# Step 1: 发起HTTP请求
url = 'https://www.zdaye.com' # 目标网址
response = requests.get(url)
# Step 2: 解析页面内容
soup = BeautifulSoup(response.content, 'html.parser')
# Step 3: 提取和处理数据
# TODO: 根据需要进行数据提取和处理,如找到特定标签、类名等
# Step 4: 输出结果
# TODO: 根据需要将处理后的数据进行输出,如写入文件或打印到控制台
```
这个模板包含了发起HTTP请求、解析页面内容、提取和处理数据以及输出结果的基本步骤。你可以根据自己的需求进行相应的修改和补充。例如,你可以使用请求头模拟浏览器访问,防止被网站阻止或限制;你也可以使用异常处理机制来捕获请求异常,并进行适当的错误处理;另外,你可以使用csv库将爬取的数据保存到CSV文件中,根据实际数据结构编写将数据写入CSV文件的相关代码。
阅读全文
相关推荐
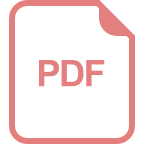
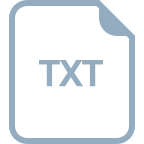
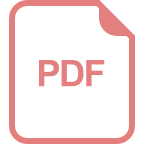
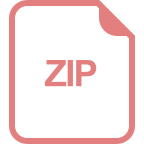
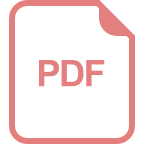
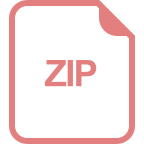
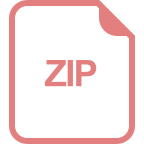
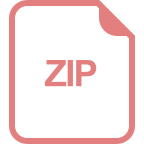
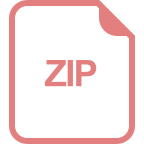
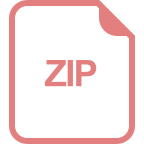
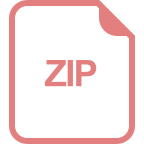
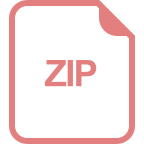
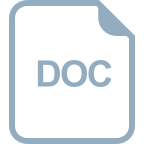
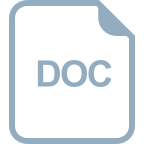
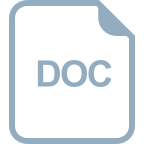
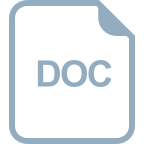
