#include <stdio.h> long fact(int n); int main() { int n, result = 0; printf("Input n:"); scanf("%d", &n); result = fact(n); printf("%d != %d", n, result); return 0; } long fact(int n) { int result; if (n < 0) printf("n<0,data error!\n"); else { result = n * fact(n - 1); return result; } }
时间: 2023-06-20 19:05:32 浏览: 134
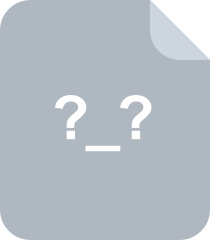
#include <stdio.h>
这段代码实现的是阶乘函数,但存在一些问题。首先,在函数fact中没有考虑到n等于0和1的情况,应该添加对这两种情况的处理。另外,当n等于0时,递归调用会一直进行下去,导致程序崩溃,应该在函数开头加上判断n是否等于0的语句,如果是则直接返回1。最后,函数返回类型应该为long,因为阶乘的结果可能很大,超过int类型的范围。
下面是修改后的代码:
```c
#include <stdio.h>
long fact(int n);
int main() {
int n, result = 0;
printf("Input n:");
scanf("%d", &n);
result = fact(n);
printf("%d! = %ld", n, result);
return 0;
}
long fact(int n) {
if (n < 0)
printf("n<0,data error!\n");
else if (n == 0 || n == 1)
return 1;
else
return n * fact(n - 1);
}
```
阅读全文
相关推荐


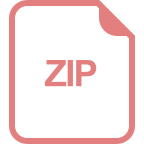












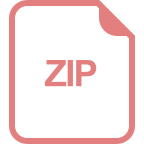
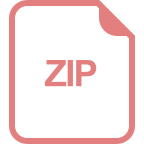
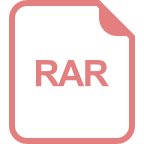