利用LSTM预测股票的python代码
时间: 2023-10-24 17:21:06 浏览: 112
对于一个AI语言模型来说,预测股票价格是一项复杂的任务。以下是一个使用LSTM模型预测股票价格的Python代码示例:
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.preprocessing import MinMaxScaler
from keras.models import Sequential
from keras.layers import Dense, LSTM
# Load the data
data = pd.read_csv('AAPL.csv', date_parser=True)
data.head()
# Plot the data
plt.figure(figsize=(16, 8))
plt.title('Close Price History')
plt.plot(data['Close'])
plt.xlabel('Date', fontsize=18)
plt.ylabel('Close Price USD ($)', fontsize=18)
plt.show()
# Prepare the data
scaler = MinMaxScaler(feature_range=(0, 1))
scaled_data = scaler.fit_transform(data['Close'].values.reshape(-1, 1))
prediction_days = 60
x_train = []
y_train = []
for x in range(prediction_days, len(scaled_data)):
x_train.append(scaled_data[x - prediction_days:x, 0])
y_train.append(scaled_data[x, 0])
x_train, y_train = np.array(x_train), np.array(y_train)
x_train = np.reshape(x_train, (x_train.shape[0], x_train.shape[1], 1))
# Build the LSTM model
model = Sequential()
model.add(LSTM(units=50, return_sequences=True, input_shape=(x_train.shape[1], 1)))
model.add(LSTM(units=50))
model.add(Dense(units=1))
model.compile(optimizer='adam', loss='mean_squared_error')
model.fit(x_train, y_train, epochs=1, batch_size=1)
# Prepare the test data
test_data = pd.read_csv('AAPL_test.csv', date_parser=True)
actual_prices = test_data['Close'].values
total_dataset = pd.concat((data['Close'], test_data['Close']), axis=0)
model_inputs = total_dataset[len(total_dataset) - len(test_data) - prediction_days:].values
model_inputs = model_inputs.reshape(-1, 1)
model_inputs = scaler.transform(model_inputs)
# Make predictions on test data
x_test = []
for x in range(prediction_days, len(model_inputs)):
x_test.append(model_inputs[x - prediction_days:x, 0])
x_test = np.array(x_test)
x_test = np.reshape(x_test, (x_test.shape[0], x_test.shape[1], 1))
predicted_prices = model.predict(x_test)
predicted_prices = scaler.inverse_transform(predicted_prices)
# Plot the predictions
plt.figure(figsize=(16, 8))
plt.title('AAPL Stock Price Prediction')
plt.plot(actual_prices, color='black', label='Actual Prices')
plt.plot(predicted_prices, color='green', label='Predicted Prices')
plt.xlabel('Time')
plt.ylabel('Price')
plt.legend()
plt.show()
```
在此代码中,我们使用了Apple公司的股票数据。首先,我们使用Pandas库加载数据,然后使用Matplotlib库绘制股票价格的历史走势图。接下来,我们使用Scikit-learn库的MinMaxScaler类来缩放数据。然后,我们将数据分为训练集和测试集,并使用LSTM模型来训练模型。最后,我们使用模型对测试集进行预测,并使用Matplotlib库绘制预测结果。
阅读全文
相关推荐
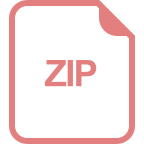
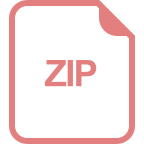
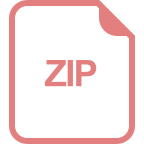
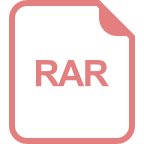
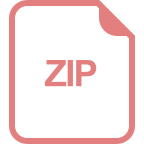
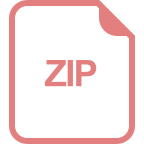
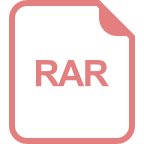
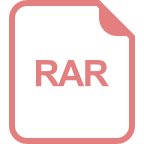
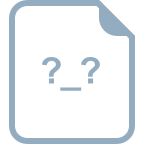
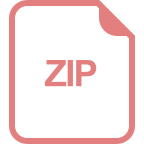
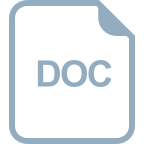
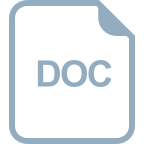
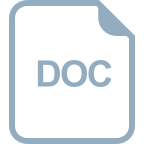
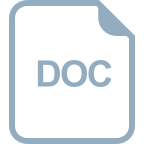
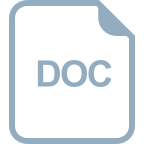

