cpp实现从cpp-httplib server下载本地文件
时间: 2024-10-25 08:16:38 浏览: 105
在C++中,你可以使用`cpp-httplib`库来实现HTTP客户端功能,包括从服务器下载文件。以下是一个简单的示例,展示如何使用这个库下载本地文件:
```cpp
#include "httplib.h"
// 下载函数
bool download_file(const std::string& url, const std::string& local_path) {
// 创建一个http客户端对象
htptplib::Client client(url.c_str(), 80);
// 发送GET请求
auto response = client.Get();
// 检查请求是否成功(状态码200表示成功)
if (response.status_code != 200) {
std::cerr << "Failed to download file: " << response.status << ": " << response.reason << std::endl;
return false;
}
// 获取响应体的长度
auto content_length = response.headers.count("Content-Length");
if (!content_length) {
std::cerr << "Missing Content-Length header" << std::endl;
return false;
}
int64_t content_length_int = std::stoll(response.headers.at("Content-Length"));
// 打开本地文件并写入数据
std::ofstream out(local_path);
if (!out) {
std::cerr << "Failed to open local file for writing" << std::endl;
return false;
}
out.seekp(0, std::ios::end); // Set the position indicator to the end of the stream
out.clear(); // Clear any error flags
// 如果内容长度大于零,逐块读取并写入文件
if (content_length_int > 0) {
char buffer[1024];
while (client.HasMore()) {
size_t read_size = client.Read(buffer, sizeof(buffer));
out.write(buffer, read_size);
}
} else { // 如果内容长度为0,说明可能是空响应头
out.write("\0", 1);
}
// 关闭连接和文件流
client.Close();
out.close();
return true;
}
// 使用示例
int main() {
std::string url = "http://example.com/file.zip";
std::string local_path = "file.zip"; // 指定本地保存路径
if (download_file(url, local_path)) {
std::cout << "Download successful!" << std::endl;
} else {
std::cout << "Download failed." << std::endl;
}
return 0;
}
```
阅读全文
相关推荐
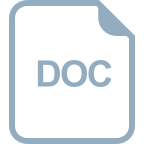
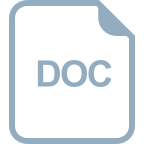
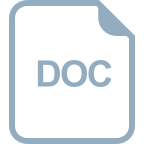


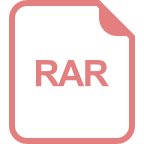
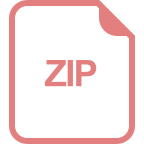

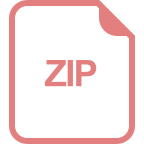
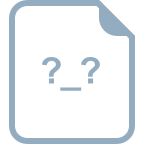
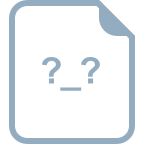
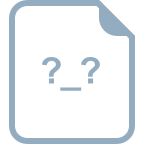
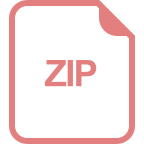




