用c plus plus 代码,基于httplib开源库实现http流式传输文件
时间: 2023-09-21 14:05:48 浏览: 307
### 回答1:
我可以提供一些建议,但是我并不是很熟悉 C++ 代码,你可以看一下 httplib 开源库的文档,里面有很多示例代码,也可以参考一些网上的教程,比如这篇文章:https://www.programmingknowledge.com/c-plus-plus/http-client-in-cpp/
### 回答2:
基于httplib开源库实现http流式传输文件的C++代码如下所示:
```cpp
#include <iostream>
#include <fstream>
#include <httplib/httplib.h>
using namespace std;
void sendFile(const httplib::Request& req, httplib::Response& res) {
std::ifstream file("file_path", std::ofstream::binary); // 替换为要传输的文件路径
if (file.fail()) {
res.status = 404;
res.set_content("Failed to open file", "text/plain");
return;
}
res.set_content_provider(
file.tellg(), // 文件大小
"application/octet-stream", // 设置文件的MIME类型
[file](size_t offset, size_t /*max_length*/, httplib::DataSink& sink) {
file.seekg(offset);
char buffer[1024];
file.read(buffer, sizeof(buffer));
size_t bytesRead = file.gcount();
if (bytesRead <= 0) {
// 文件传输结束
return -1;
} else {
sink.write(buffer, bytesRead);
return bytesRead;
}
}
);
}
int main() {
httplib::Server svr;
svr.Get("/download", sendFile);
svr.listen("localhost", 8080); // 设置监听地址和端口
return 0;
}
```
请注意,上述代码中的"file_path"需要替换为实际要传输的文件的路径。代码使用httplib库创建了一个本地服务器,提供了一个GET请求的路由"/download"以供文件下载。使用set_content_provider()方法设置文件传输的内容提供者,从文件读取数据并传输到客户端。
运行代码后,您可以通过在浏览器中访问"http://localhost:8080/download"来下载文件。
### 回答3:
使用C++代码基于httplib开源库实现HTTP流式传输文件可以通过以下步骤进行:
1. 包含httplib库的头文件:`#include <httplib.h>`
2. 创建一个HTTP Client对象:`httplib::Client client;`
3. 指定要传输的文件路径和URL:`std::string filepath = "path/to/file";`和`std::string url = "http://example.com/upload";`
4. 打开要传输的文件:`std::ifstream file(filepath, std::ios::binary);`
5. 使用httplib库的POST方法发送文件数据到服务器:`auto res = client.Post(url.c_str(), file);`
6. 检查是否成功发送文件:`if (res && res->status == 200)`
7. 关闭文件:`file.close();`
完成上述步骤后,你就成功地使用C++代码基于httplib开源库实现了HTTP流式传输文件。
需要注意的是,以上代码仅用于传输文件,如果想要实现进度条或在传输期间进行其他操作,需要结合其他库或自行实现。另外,确保你已将httplib库正确地包含到你的项目中。
相关推荐
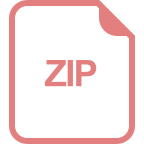














