c++语言顺序表基本操作
时间: 2023-10-15 21:06:43 浏览: 96
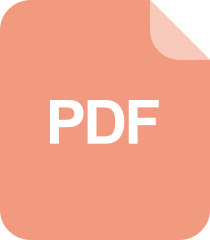
c语言实现顺序表的基本操作

引用是一段C++代码,引用和引用是关于线性表的一些操作。c语言顺序表的基本操作包括初始化线性表、销毁线性表、清空线性表、判断线性表是否为空、获取线性表长度、获取线性表指定位置的元素、修改线性表指定位置的元素、在线性表指定位置插入元素、删除线性表指定位置的元素。这些基本操作可以通过调用对应的函数来实现。相关问题如下:
相关问题:
1. 如何初始化一个c语言顺序表?
2. 如何判断一个c语言顺序表是否为空?
3. 如何获取一个c语言顺序表的长度?
4. 如何在一个c语言顺序表的指定位置插入元素?
5. 如何删除一个c语言顺序表的指定位置的元素?
阅读全文
相关推荐
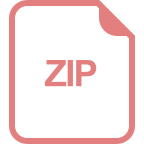
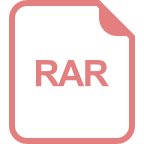
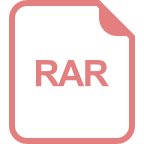
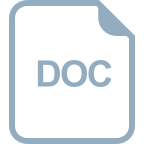
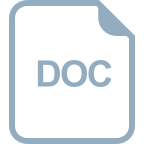
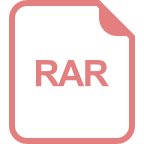
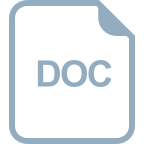
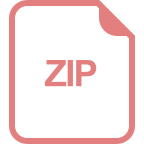
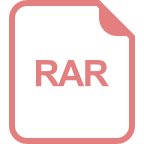
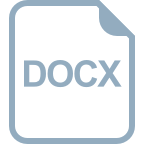
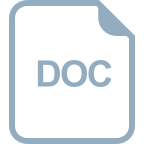
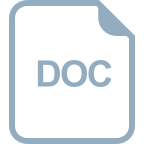
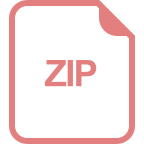
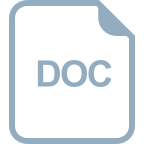
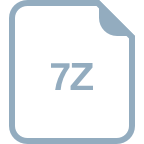
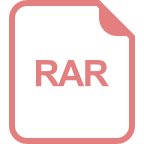