链队列的基本操作c++
时间: 2023-12-06 16:02:37 浏览: 51
链队列是通过链表实现的队列,链队列的基本操作包括:
1. 初始化:初始化一个空的链队列。
2. 入队:将一个元素插入队列尾部。
3. 出队:删除队头元素,并返回其值。
4. 取队头元素:返回队头元素的值。
5. 判空:判断链队列是否为空。
6. 遍历:按顺序输出队列中的所有元素。
以下是链队列的基本操作实现的 C 语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 链队列结点的结构体
struct Node {
int data;
struct Node *next;
};
// 链队列的结构体
typedef struct {
struct Node *front; // 队头指针
struct Node *rear; // 队尾指针
} LinkQueue;
// 初始化链队列
void initQueue(LinkQueue *Q) {
Q->front = Q->rear = (struct Node *) malloc(sizeof(struct Node));
Q->front->next = NULL;
}
// 判断链队列是否为空
int isEmpty(LinkQueue *Q) {
return Q->front == Q->rear;
}
// 元素入队
void enQueue(LinkQueue *Q, int x) {
struct Node *newNode = (struct Node *) malloc(sizeof(struct Node));
newNode->data = x;
newNode->next = NULL;
Q->rear->next = newNode;
Q->rear = newNode;
}
// 元素出队
int deQueue(LinkQueue *Q) {
if (isEmpty(Q)) {
printf("队列已空,不能删除元素!\n");
exit(1);
} else {
struct Node *p = Q->front->next;
int x = p->data;
Q->front->next = p->next;
if (Q->rear == p) {
Q->rear = Q->front;
}
free(p);
return x;
}
}
// 取队头元素
int getFront(LinkQueue *Q) {
if (isEmpty(Q)) {
printf("队列已空,没有队头元素!\n");
exit(1);
} else {
return Q->front->next->data;
}
}
// 遍历链队列
void traverseQueue(LinkQueue *Q) {
struct Node *p = Q->front->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
LinkQueue Q;
initQueue(&Q);
enQueue(&Q, 1);
enQueue(&Q, 2);
enQueue(&Q, 3);
enQueue(&Q, 4);
printf("遍历队列:");
traverseQueue(&Q);
printf("队头元素:%d\n", getFront(&Q));
printf("出队元素:%d\n", deQueue(&Q));
printf("出队元素:%d\n", deQueue(&Q));
printf("遍历队列:");
traverseQueue(&Q);
return 0;
}
```
相关推荐
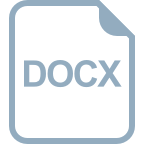
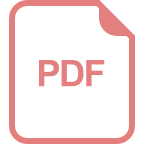














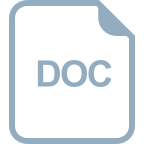