yolov8代码讲解
时间: 2023-10-04 19:12:05 浏览: 123
Yolov8 是一种目标检测算法,它是基于深度学习框架 Darknet 实现的。下面是 Yolov8 的代码讲解:
首先,引入必要的库和模块:
```python
import cv2
import numpy as np
from darknet import Darknet
```
然后,定义一个函数来加载 Yolov8 模型:
```python
def load_yolov8_model(config_file, weight_file):
model = Darknet(config_file)
model.load_weights(weight_file)
return model
```
接下来,定义一个函数用于进行目标检测:
```python
def detect_objects(image, model, class_names, confidence_threshold, nms_threshold):
# 图像处理
img = cv2.resize(image, (model.width, model.height))
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
img = np.transpose(img, (2, 0, 1))
img = img.astype(np.float32) / 255.0
img = np.expand_dims(img, axis=0)
# 模型推理
model.eval()
with torch.no_grad():
input_img = torch.from_numpy(img)
if torch.cuda.is_available():
input_img = input_img.cuda()
model.cuda()
output = model(input_img)
# 解析检测结果
detections = output[0]
detections = non_max_suppression(detections, confidence_threshold, nms_threshold)
# 绘制检测框和标签
for detection in detections:
x, y, w, h = detection[0:4] * image.shape[1]
class_id = int(detection[6])
label = class_names[class_id]
confidence = detection[4]
cv2.rectangle(image, (int(x - w / 2), int(y - h / 2)), (int(x + w / 2), int(y + h / 2)), (255, 0, 0), 2)
cv2.putText(image, f'{label}: {confidence}', (int(x - w / 2), int(y - h / 2 - 10)), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (255, 0, 0), 2)
return image
```
最后,使用上述函数对图像进行目标检测:
```python
# 加载模型
model = load_yolov8_model('yolov8.cfg', 'yolov8.weights')
# 定义类别名称
class_names = ['person', 'car', 'cat', ...]
# 加载图像
image = cv2.imread('image.jpg')
# 进行目标检测
result_image = detect_objects(image, model, class_names, confidence_threshold=0.5, nms_threshold=0.4)
# 显示结果
cv2.imshow('Result', result_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这就是使用 Yolov8 进行目标检测的代码讲解。注意,以上代码仅为示例,实际应用中可能需要根据具体情况进行调整和优化。
阅读全文
相关推荐
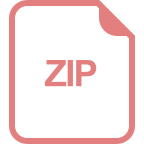
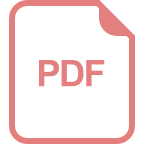










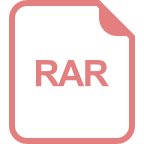




