Image.open()函数用于打开并读取给定的图像文件,返回一个Image对象,如何编程查看image对象内容
时间: 2024-10-11 15:07:10 浏览: 30
`Image.open()`函数确实用于打开图像文件,并返回一个`Image`对象,这是PIL库中的一个核心类。要查看`Image`对象的内容,通常有几种方式:
1. **元数据检查**:你可以通过访问`Image`对象的属性获取一些基本信息,比如宽度、高度、模式(颜色通道数)等。例如:
```python
width, height = map.size
mode = map.mode
```
2. **预览图像**:可以使用`imshow()`函数来自matplotlib,这将直接显示图像的缩略图:
```python
plt.imshow(map)
plt.show()
```
注意:在显示之前,可能需要先转换图像到适合matplotlib格式,例如RGB。
3. **打印像素值**:如果你想看每个像素的具体颜色,可以遍历图像矩阵,例如:
```python
for x in range(width):
for y in range(height):
pixel_color = map.getpixel((x, y))
print(pixel_color)
```
然而,这种方法可能会因为屏幕或字符限制而显得不便。
4. **分析元数据**:对于更复杂的元数据,如EXIF信息,可以使用`getexif()`方法。
以上操作取决于你想要了解图像的哪种详细程度。如果你只是想快速预览,那么预览和元数据检查是最常用的。
相关问题
class COCODataset(Dataset): def __init__(self, root_dir, transform=None): self.root_dir = root_dir self.transform = transform self.image_files = os.listdir(root_dir) def __len__(self): return len(self.image_files) def __getitem__(self, idx): img_path = os.path.join(self.root_dir, self.image_files[idx]) image = Image.open(img_path).convert('RGB') if self.transform: image = self.transform(image) return image
这段代码是一个 PyTorch 中的 Dataset 类,用于加载 COCO 数据集中的图像数据。具体来说,构造函数中接收两个参数:数据集根目录 root_dir 和可选的数据预处理函数 transform。在初始化过程中,该类读取指定目录下的所有图像文件名,并保存在 image_files 中。__len__ 方法返回数据集大小,即图像数量。__getitem__ 方法根据给定的索引 idx 加载对应的图像数据,并将其转换为 RGB 格式。如果指定了 transform 函数,则在返回数据前进行数据预处理操作。最终,该方法返回处理后的图像数据。该类可以用于 PyTorch 的 DataLoader 中,以便进行批量训练和数据增强。
from skimage.segmentation import slic, mark_boundaries import torchvision.transforms as transforms import numpy as np from PIL import Image import matplotlib.pyplot as plt # 加载图像 image = Image.open('3.jpg') # 转换为 PyTorch 张量 transform = transforms.ToTensor() img_tensor = transform(image).unsqueeze(0) # 将 PyTorch 张量转换为 Numpy 数组 img_np = img_tensor.numpy().transpose(0, 2, 3, 1)[0] # 使用 SLIC 算法生成超像素标记图 segments = slic(img_np, n_segments=60, compactness=10) # 可视化超像素索引映射 plt.imshow(segments, cmap='gray') plt.show() # 将超像素索引映射可视化 segment_img = mark_boundaries(img_np, segments) # 将 Numpy 数组转换为 PIL 图像 segment_img = Image.fromarray((segment_img * 255).astype(np.uint8)) # 保存超像素索引映射可视化 segment_img.save('segment_map.jpg') 将上述代码中引入超像素池化代码:import cv2 import numpy as np # 读取图像 img = cv2.imread('3.jpg') # 定义超像素分割器 num_segments = 60 # 超像素数目 slic = cv2.ximgproc.createSuperpixelSLIC(img, cv2.ximgproc.SLICO, num_segments) # 进行超像素分割 slic.iterate(10) # 获取超像素标签和数量 labels = slic.getLabels() num_label = slic.getNumberOfSuperpixels() # 对每个超像素进行池化操作,这里使用平均值池化 pooled = [] for i in range(num_label): mask = labels == i region = img[mask] pooled.append(region.mean(axis=0)) # 将池化后的特征图可视化 pooled = np.array(pooled, dtype=np.uint8) pooled_features = pooled.reshape(-1) pooled_img = cv2.resize(pooled_features, (img.shape[1], img.shape[0]), interpolation=cv2.INTER_NEAREST) print(pooled_img.shape) cv2.imshow('Pooled Image', pooled_img) cv2.waitKey(0),并显示超像素池化后的特征图
好的,这个问题是要求你在给定的代码中添加超像素池化的实现,并显示超像素池化后的特征图。以下是添加超像素池化的代码和显示超像素池化后的特征图的代码:
```
import cv2
import numpy as np
# 读取图像
img = cv2.imread('3.jpg')
# 定义超像素分割器
num_segments = 60 # 超像素数目
slic = cv2.ximgproc.createSuperpixelSLIC(img, cv2.ximgproc.SLICO, num_segments)
# 进行超像素分割
slic.iterate(10)
# 获取超像素标签和数量
labels = slic.getLabels()
num_label = slic.getNumberOfSuperpixels()
# 对每个超像素进行池化操作,这里使用平均值池化
pooled = []
for i in range(num_label):
mask = labels == i
region = img[mask]
pooled.append(region.mean(axis=0))
# 将池化后的特征图可视化
pooled = np.array(pooled, dtype=np.uint8)
pooled_features = pooled.reshape(-1)
pooled_img = cv2.resize(pooled_features, (img.shape[1], img.shape[0]), interpolation=cv2.INTER_NEAREST)
# 显示超像素池化后的特征图
cv2.imshow('Pooled Image', pooled_img.reshape(img.shape))
cv2.waitKey(0)
```
注意,这里使用的是 OpenCV 库中的超像素分割器,并且超像素池化使用的是平均值池化。最后,使用 OpenCV 库中的 imshow() 函数和 waitKey() 函数来显示超像素池化后的特征图。
阅读全文
相关推荐
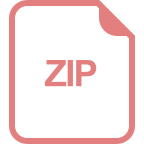
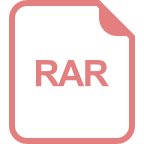
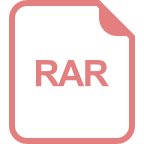
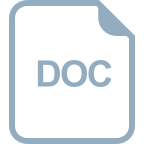
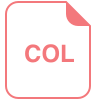
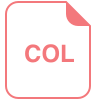
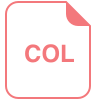









