帮我使用c语言编写一段程序,实现从一个bin文件中读取数据,放入一个定义为int a[8]的数组中,每次读取完sizeof(a)后打印出来,然后开始下一次读取,循环读取三次。
时间: 2024-12-24 21:38:19 浏览: 8
当然可以,下面是一个简单的C语言程序,它会从二进制文件中逐块读取数据并存储到`int a[8]`数组中,每读取完数组大小就打印内容。这个程序假设二进制文件是以整数的形式连续存储的。
```c
#include <stdio.h>
#include <fcntl.h> // for open and close file descriptors
#include <unistd.h> // for read
#define ARRAY_SIZE 8
// 函数用于读取文件并填充数组
void readBinFile(char *filename, int arr[ARRAY_SIZE]) {
int fd = open(filename, O_RDONLY); // 打开文件
if (fd == -1) {
perror("Error opening file");
return;
}
off_t bytes_read = 0;
while (bytes_read < sizeof(arr)) { // 循环读取直到读满数组
ssize_t num_bytes = read(fd, arr + bytes_read, sizeof(arr) - bytes_read);
if (num_bytes <= 0) {
perror("Error reading from file");
break;
}
bytes_read += num_bytes; // 更新已读字节数
// 每次读取完数组大小就打印内容
printf("Read data: ");
for (int i = 0; i < ARRAY_SIZE && bytes_read > i; i++) {
printf("%d ", arr[i]);
}
printf("\n");
// 如果未读完整个数组,继续读取
}
close(fd); // 关闭文件描述符
}
int main() {
int a[ARRAY_SIZE];
char filename[] = "example.bin"; // 替换为你实际的二进制文件名
readBinFile(filename, a);
return 0;
}
```
**相关问题--:**
1. 这段代码对文件的错误处理是如何进行的?
2. 如果文件中的数据不是按照整数形式存储,这段代码还能正常工作吗?
3. 该程序如何修改才能处理大于8个元素的大数组?
阅读全文
相关推荐
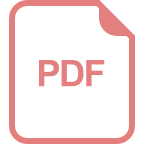
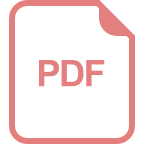
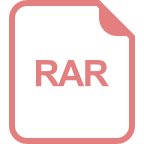















