static char *TCPCommand; static pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER; int SetUpTCPtoSendInformation(char option[], char **command) { static int isFirstCall = 1; pthread_mutex_lock(&mutex); // 加锁 if (isFirstCall && strstr(option, "set")) { if (TCPCommand != NULL) { free(TCPCommand); } TCPCommand = malloc(strlen(*command) + 1); if (TCPCommand == NULL) { printf("Failed to set and obtain TCP command variable memory allocation\n"); goto fail; } strcpy(TCPCommand, *command); printf("set:%s\n", TCPCommand); isFirstCall = 0; goto succeed; } else if (!isFirstCall && strstr(option, "get") && TCPCommand != NULL && strlen(TCPCommand)) { free(*command); *command = malloc(strlen(TCPCommand) + 1); strcpy(*command, TCPCommand); printf("get:%s\n", *command); memset(TCPCommand, '\0', strlen(TCPCommand)); free(TCPCommand); TCPCommand = NULL; isFirstCall = 1; goto succeed; } memset(*command, 0, sizeof(*command)); strcpy(*command, ""); fail: pthread_mutex_unlock(&mutex); // 解锁 return 0; succeed: pthread_mutex_unlock(&mutex); // 解锁 return 1; } SetUpTCPtoSendInformation("set","sssssss"); char *resultbuf =NULL; SetUpTCPtoSendInformation("get",&resultbuf); 这样子调用多么如果不对请帮我修改函数或者调用方法
时间: 2024-04-20 08:22:41 浏览: 52
您的函数调用看起来是正确的。它首先调用 `SetUpTCPtoSendInformation` 函数,传递了参数 `"set"` 和一个字符串指针 `&resultbuf`。在该函数内部,它会将 `TCPCommand` 设置为传递的命令,并在成功时打印出来。
然后,它再次调用 `SetUpTCPtoSendInformation` 函数,传递了参数 `"get"` 和字符串指针 `&resultbuf`。在该函数内部,它会将 `TCPCommand` 的值复制到 `resultbuf` 中,并在成功时打印出来。
根据您的代码,函数应该正常工作。请注意,`resultbuf` 需要在调用 `SetUpTCPtoSendInformation` 函数之前初始化为 `NULL`。此外,由于函数内部使用了互斥锁,所以确保在多线程环境下使用时不会出现竞态条件。
如果您有其他问题或需要进一步的帮助,请随时告诉我。
相关问题
static char *TCPCommand; static pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER; int SetUpTCPtoSendInformation(char option[], char *command) { pthread_mutex_lock(&mutex); // 加锁 if (strstr(option, "set")) { TCPCommand = malloc(strlen(command) + 1); if (TCPCommand == NULL) { CON_LOG("Failed to set and obtain TCP command variable memory allocation"); goto fail; } strcpy(TCPCommand, command); goto succeed; } else if (strstr(option, "get") && TCPCommand != NULL && strlen(TCPCommand)) { strcpy(command, TCPCommand); memset(TCPCommand, '\0', strlen(TCPCommand)); free(TCPCommand); pthread_mutex_unlock(&mutex); // 解锁 goto succeed; } memset(command, 0, sizeof(command)); strcpy(command, ""); fail: pthread_mutex_unlock(&mutex); // 解锁 return 0; succeed: pthread_mutex_unlock(&mutex); // 解锁 return 1; } 编写一个多进程或多进程调用这个函数
您可以使用多线程来调用`SetUpTCPtoSendInformation`函数。以下是一个示例代码,其中使用了两个线程来调用该函数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
static char *TCPCommand;
static pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
int SetUpTCPtoSendInformation(char option[], char *command) {
pthread_mutex_lock(&mutex); // 加锁
if (strstr(option, "set")) {
TCPCommand = malloc(strlen(command) + 1);
if (TCPCommand == NULL) {
printf("Failed to set and obtain TCP command variable memory allocation\n");
goto fail;
}
strcpy(TCPCommand, command);
goto succeed;
} else if (strstr(option, "get") && TCPCommand != NULL && strlen(TCPCommand)) {
strcpy(command, TCPCommand);
memset(TCPCommand, '\0', strlen(TCPCommand));
free(TCPCommand);
pthread_mutex_unlock(&mutex); // 解锁
goto succeed;
}
memset(command, 0, sizeof(command));
strcpy(command, "");
fail:
pthread_mutex_unlock(&mutex); // 解锁
return 0;
succeed:
pthread_mutex_unlock(&mutex); // 解锁
return 1;
}
void* thread_func(void* arg) {
char option[4];
char command[100];
strcpy(option, "set");
strcpy(command, "Example Command");
SetUpTCPtoSendInformation(option, command);
return NULL;
}
int main() {
pthread_t thread1, thread2;
pthread_create(&thread1, NULL, thread_func, NULL);
pthread_create(&thread2, NULL, thread_func, NULL);
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
return 0;
}
```
在上面的示例中,`thread_func`函数作为线程的入口点,在每个线程中调用`SetUpTCPtoSendInformation`函数。通过使用`pthread_create`函数创建两个线程,并使用`pthread_join`函数等待线程的完成。
请注意,由于使用了全局变量和互斥锁,线程将共享`TCPCommand`变量,并且在访问和修改该变量时需要进行互斥操作。
static char *TCPCommand = NULL; static pthread_mutex_t mutex = P_MUTEX_INITIALIZER; int SetTCPCommand(char *command) { pthread_mutex_lock(&mutex); // 加锁 if (TCPCommand != NULL) { free(TCPCommand); } TCPCommand = malloc(strlen(command) + 1); if (TCPCommand == NULL) { printf("Failed to allocate memory for TCP command\n"); pthread_mutex_unlock(&mutex); // 解锁 return 0; } strcpy(TCPCommand, command); printf("set: %s\n", TCPCommand); pthread_mutex_unlock(&mutex); // 解锁 return 1; } int GetTCPCommand(char **command) { pthread_mutex_lock(&mutex); // 加锁 if (TCPCommand == NULL || strlen(TCPCommand) == 0) { printf("TCP command is empty\n"); pthread_mutex_unlock(&mutex); // 解锁 return 0; } *command = malloc(strlen(TCPCommand) + 1); if (*command == NULL) { printf("Failed to allocate memory for command\n"); pthread_mutex_unlock(&mutex); // 解锁 return 0; } strcpy(*command, TCPCommand); printf("get: %s\n", *command); free(TCPCommand); TCPCommand = NULL; pthread_mutex_unlock(&mutex); // 解锁 return 1; }根据这个帮我优化代码,因为我是在另一个进程一直执行GetTCPCommand,所以他会先于SetTCPCommand执行
根据你的描述,如果另一个进程在不断执行`GetTCPCommand`,而且在调用`SetTCPCommand`之前执行了`GetTCPCommand`,那么可能会出现问题。这是因为在获取到TCPCommand之后,你立即将其释放,并将其置为NULL,这样在另一个进程中再次调用`GetTCPCommand`时就会返回空值。
为了解决这个问题,你可以使用条件变量来实现等待和通知机制。以下是修改后的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <pthread.h>
static char *TCPCommand = NULL;
static pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
static pthread_cond_t cond = PTHREAD_COND_INITIALIZER;
int SetTCPCommand(char *command) {
pthread_mutex_lock(&mutex); // 加锁
if (TCPCommand != NULL) {
free(TCPCommand);
}
TCPCommand = malloc(strlen(command) + 1);
if (TCPCommand == NULL) {
printf("Failed to allocate memory for TCP command\n");
pthread_mutex_unlock(&mutex); // 解锁
return 0;
}
strcpy(TCPCommand, command);
printf("set: %s\n", TCPCommand);
pthread_cond_signal(&cond); // 发送信号通知等待的线程
pthread_mutex_unlock(&mutex); // 解锁
return 1;
}
int GetTCPCommand(char **command) {
pthread_mutex_lock(&mutex); // 加锁
while (TCPCommand == NULL || strlen(TCPCommand) == 0) {
// 等待条件满足
pthread_cond_wait(&cond, &mutex);
}
*command = malloc(strlen(TCPCommand) + 1);
if (*command == NULL) {
printf("Failed to allocate memory for command\n");
pthread_mutex_unlock(&mutex); // 解锁
return 0;
}
strcpy(*command, TCPCommand);
printf("get: %s\n", *command);
free(TCPCommand);
TCPCommand = NULL;
pthread_mutex_unlock(&mutex); // 解锁
return 1;
}
```
在修改后的代码中,我们引入了一个条件变量`cond`来实现等待和通知机制。在`GetTCPCommand`函数中,如果TCPCommand为空,则调用`pthread_cond_wait`等待条件满足。而在`SetTCPCommand`函数中,我们在设置完TCPCommand并释放锁之前,调用`pthread_cond_signal`发送信号通知等待的线程。
这样,在另一个进程一直执行`GetTCPCommand`时,如果在调用`SetTCPCommand`之前执行了`GetTCPCommand`,它会等待直到有新的TCPCommand被设置,并且不会错过通知。
希望这次能满足你的需求!如果你还有其他问题,请随时提问。
阅读全文
相关推荐
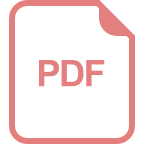
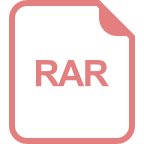
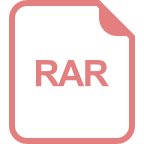












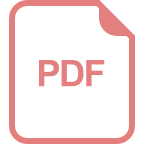
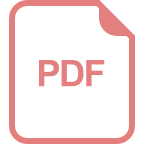