int SetUpTCPtoSendInformation(char option[], char *command) { static char *TCPCommand; static pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER; static int isFirstCall = 1; pthread_mutex_lock(&mutex); // 加锁 if (isFirstCall && strstr(option, "set")) { TCPCommand = malloc(strlen(command) + 1); if (TCPCommand == NULL) { CON_LOG("Failed to set and obtain TCP command variable memory allocation"); goto fail; } strcpy(TCPCommand, command); isFirstCall = 0; goto succeed; } else if (!isFirstCall && strstr(option, "get") && TCPCommand != NULL && strlen(TCPCommand)) { strcpy(command, TCPCommand); memset(TCPCommand, '\0', strlen(TCPCommand)); free(TCPCommand); isFirstCall = 1; goto succeed; } memset(command, 0, sizeof(command)); strcpy(command, ""); fail: pthread_mutex_unlock(&mutex); // 解锁 return 0; succeed: pthread_mutex_unlock(&mutex); // 解锁 return 1; }这样呢
时间: 2024-04-08 15:34:13 浏览: 23
您提供的代码已经进行了必要的修改,看起来是可以正常工作的。它使用了静态变量`TCPCommand`和`isFirstCall`来跟踪第一次调用和后续调用,并使用互斥锁确保线程安全性。
我注意到,您使用了`goto`语句。虽然在某些情况下可以使用`goto`语句,但它们可能会使代码变得难以理解和维护。您可以考虑使用更传统的控制流结构(如`if-else`语句)来替代。
以下是没有使用`goto`语句的修改版本:
```c
int SetUpTCPtoSendInformation(char option[], char *command) {
static char *TCPCommand;
static pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
static int isFirstCall = 1;
pthread_mutex_lock(&mutex); // 加锁
int result = 0; // 默认返回失败
if (isFirstCall && strstr(option, "set")) {
TCPCommand = malloc(strlen(command) + 1);
if (TCPCommand == NULL) {
CON_LOG("Failed to set and obtain TCP command variable memory allocation");
} else {
strcpy(TCPCommand, command);
isFirstCall = 0;
result = 1; // 设置成功,返回成功
}
} else if (!isFirstCall && strstr(option, "get") && TCPCommand != NULL && strlen(TCPCommand)) {
strcpy(command, TCPCommand);
memset(TCPCommand, '\0', strlen(TCPCommand));
free(TCPCommand);
TCPCommand = NULL;
isFirstCall = 1;
result = 1; // 获取成功,返回成功
} else {
memset(command, 0, sizeof(command));
strcpy(command, "");
}
pthread_mutex_unlock(&mutex); // 解锁
return result;
}
```
请注意,这只是一种修改方法,您可以根据自己的需求进行进一步调整。
相关推荐
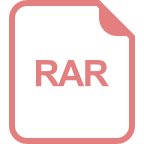
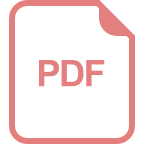
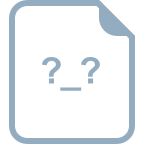














