c++的A*算法伪代码
时间: 2023-12-04 14:40:28 浏览: 34
以下是C++的A*算法伪代码:
```c++
// 定义节点结构体
struct Node {
int x, y; // 节点坐标
int g, h; // g为起点到该点的实际代价,h为该点到终点的估计代价
int f; // f = g + h
bool operator<(const Node& other) const {
return f > other.f; // 重载小于号,用于优先队列排序
}
};
// A*算法
int A_star(Node start, Node end) {
priority_queue<Node> pq; // 优先队列,用于存储待扩展的节点
unordered_map<int, unordered_map<int, bool>> visited; // 哈希表,用于记录节点是否已经被访问过
pq.push(start); // 将起点加入优先队列
visited[start.x][start.y] = true; // 标记起点已经被访问过
while (!pq.empty()) {
Node cur = pq.top(); // 取出f值最小的节点
pq.pop();
if (cur.x == end.x && cur.y == end.y) { // 如果当前节点是终点,返回起点到终点的代价
return cur.g;
}
for (int i = 0; i < 4; i++) { // 遍历当前节点的四个相邻节点
int nx = cur.x + dx[i], ny = cur.y + dy[i]; // 计算相邻节点的坐标
if (nx < 0 || nx >= n || ny < 0 || ny >= m) { // 如果相邻节点越界,跳过
continue;
}
if (visited[nx][ny]) { // 如果相邻节点已经被访问过,跳过
continue;
}
int ng = cur.g + 1; // 计算起点到相邻节点的实际代价
int nh = heuristic(nx, ny, end.x, end.y); // 计算相邻节点到终点的估计代价
int nf = ng + nh; // 计算相邻节点的f值
pq.push({nx, ny, ng, nh, nf}); // 将相邻节点加入优先队列
visited[nx][ny] = true; // 标记相邻节点已经被访问过
}
}
return -1; // 如果无法到达终点,返回-1
}
```
相关推荐
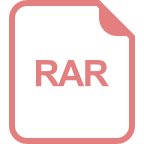














