读出文件是sfile.txt中的内容,反序写入另一个文件dfile.txt中去
时间: 2024-04-12 14:26:35 浏览: 178
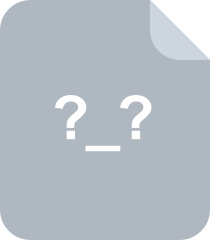
讲txt文件中的内容处理后写入另一个文件
可以使用 C 语言的文件操作函数来实现读取和反序写入文件的功能。下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
int main() {
FILE *sourceFile, *destinationFile;
char sourceFilename[] = "sfile.txt";
char destinationFilename[] = "dfile.txt";
char line[MAX_SIZE];
int numOfLines = 0;
// 打开源文件
sourceFile = fopen(sourceFilename, "r");
if (sourceFile == NULL) {
printf("无法打开源文件 %s\n", sourceFilename);
return 1;
}
// 计算源文件的行数
while (fgets(line, MAX_SIZE, sourceFile) != NULL) {
numOfLines++;
}
// 创建动态数组保存源文件的内容
char **content = (char **)malloc(numOfLines * sizeof(char *));
if (content == NULL) {
printf("内存分配失败\n");
return 1;
}
// 回到文件开始位置
rewind(sourceFile);
// 读取源文件的内容并保存到动态数组中
int i = 0;
while (fgets(line, MAX_SIZE, sourceFile) != NULL) {
line[strcspn(line, "\n")] = '\0'; // 移除换行符
content[i] = strdup(line); // 复制字符串到动态数组
i++;
}
// 关闭源文件
fclose(sourceFile);
// 打开目标文件以写入反序内容
destinationFile = fopen(destinationFilename, "w");
if (destinationFile == NULL) {
printf("无法创建目标文件 %s\n", destinationFilename);
return 1;
}
// 反序写入目标文件
for (int j = numOfLines - 1; j >= 0; j--) {
fprintf(destinationFile, "%s\n", content[j]);
}
// 关闭目标文件
fclose(destinationFile);
// 释放动态数组内存
for (int k = 0; k < numOfLines; k++) {
free(content[k]);
}
free(content);
printf("已将源文件 %s 的内容反序写入目标文件 %s\n", sourceFilename, destinationFilename);
return 0;
}
```
在上述示例代码中,我们首先打开源文件,计算源文件的行数,并创建一个动态数组来保存源文件的内容。然后,我们回到文件开始位置,将源文件的内容读取到动态数组中。接下来,我们打开目标文件,并从动态数组的末尾开始反序写入内容。最后,关闭文件并释放动态数组的内存。
请确保在运行代码之前,已经创建了名为 "sfile.txt" 的源文件。运行代码后,将会生成名为 "dfile.txt" 的目标文件,其中包含了源文件内容的反序排列。
阅读全文
相关推荐




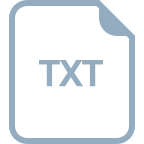
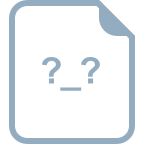
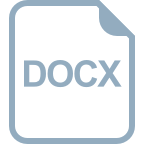
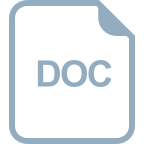








