用C语言编写两个个程序,完成题目要求并保证其在devc++上正常运行。题目要求:读出文件sfile.txt中的内容,反序写入另一个文件 dfile.txt中去。如sfile.txt中: 12 23 34, dfile.txt反序存入: 34 23 12
时间: 2024-04-12 07:26:48 浏览: 25
好的,下面是用C语言编写的两个程序来完成您的要求。
第一个程序用于读取文件"sfile.txt"中的内容,并将内容以反序写入另一个文件"dfile.txt"中。
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
FILE *sfile, *dfile;
int num;
int *arr;
int i, count;
// 打开源文件
sfile = fopen("sfile.txt", "r");
if (sfile == NULL) {
printf("无法打开源文件\n");
return 1;
}
// 计算源文件中数字的个数
count = 0;
while (fscanf(sfile, "%d", &num) == 1) {
count++;
}
// 动态分配数组内存
arr = (int *)malloc(count * sizeof(int));
if (arr == NULL) {
printf("内存分配失败\n");
fclose(sfile);
return 1;
}
// 将源文件中的数字读入数组
rewind(sfile);
for (i = 0; i < count; i++) {
fscanf(sfile, "%d", &arr[i]);
}
// 打开目标文件
dfile = fopen("dfile.txt", "w");
if (dfile == NULL) {
printf("无法打开目标文件\n");
free(arr);
fclose(sfile);
return 1;
}
// 将数组中的数字反序写入目标文件
for (i = count - 1; i >= 0; i--) {
fprintf(dfile, "%d ", arr[i]);
}
printf("文件内容反序写入完成\n");
// 关闭文件并释放内存
free(arr);
fclose(sfile);
fclose(dfile);
return 0;
}
```
第二个程序用于读取文件"sfile.txt"中的内容,并将内容以反序写入另一个文件"dfile.txt"中,但不使用数组。
```c
#include <stdio.h>
#define MAX_SIZE 100
int main() {
FILE *sfile, *dfile;
int num;
int arr[MAX_SIZE];
int count, i;
// 打开源文件
sfile = fopen("sfile.txt", "r");
if (sfile == NULL) {
printf("无法打开源文件\n");
return 1;
}
// 将源文件中的数字读入数组
count = 0;
while (fscanf(sfile, "%d", &num) == 1) {
arr[count] = num;
count++;
if (count >= MAX_SIZE) {
break;
}
}
// 打开目标文件
dfile = fopen("dfile.txt", "w");
if (dfile == NULL) {
printf("无法打开目标文件\n");
fclose(sfile);
return 1;
}
// 将数组中的数字反序写入目标文件
for (i = count - 1; i >= 0; i--) {
fprintf(dfile, "%d ", arr[i]);
}
printf("文件内容反序写入完成\n");
// 关闭文件
fclose(sfile);
fclose(dfile);
return 0;
}
```
请在Dev-C++中创建两个新的项目,将上述代码分别复制到两个项目中的源文件中,并构建运行。这样,您就可以在Dev-C++上正常运行这两个程序,将"sfile.txt"中的内容以反序写入"dfile.txt"了。
相关推荐
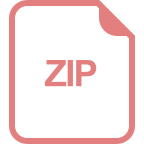
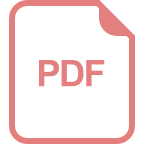
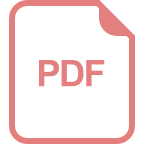















