用c语言编写程序: 1) 定义一个全局变量count,创建两个线程1和2; 2) 线程1读取全局变量count的值到局部变量a,局部变量a加10后重新赋值给count; 3)线程2读取全局变量count的值到局部变量b,局部变量b加20后重新赋值给count。
时间: 2024-09-29 08:03:17 浏览: 38
在C语言中,我们可以使用`pthread.h`库来创建和管理线程,并通过`*volatile`关键字来处理共享数据的可见性。这里是一个简单的示例代码,展示了如何按照你的需求实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
// 全局变量
volatile int count = 0;
void *thread1(void *arg) {
int a;
pthread_mutex_lock(&mutex); // 使用互斥锁防止竞态条件
a = count; // 读取count
a += 10;
count = a; // 更新count
printf("Thread 1: Count is now %d\n", count);
pthread_mutex_unlock(&mutex);
return NULL;
}
void *thread2(void *arg) {
int b;
pthread_mutex_lock(&mutex); // 获取锁
b = count; // 读取count
b += 20;
count = b; // 更新count
printf("Thread 2: Count is now %d\n", count);
pthread_mutex_unlock(&mutex);
return NULL;
}
int main() {
pthread_t thread1_id, thread2_id;
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER; // 初始化互斥锁
if (pthread_create(&thread1_id, NULL, thread1, NULL)) {
perror("Error creating thread 1");
exit(1);
}
if (pthread_create(&thread2_id, NULL, thread2, NULL)) {
perror("Error creating thread 2");
exit(1);
}
pthread_join(thread1_id, NULL);
pthread_join(thread2_id, NULL);
printf("Final count: %d\n", count);
return 0;
}
```
在这个例子中,我们首先定义了一个全局变量`count`并初始化为0。然后,我们创建了两个线程,每个线程都会先获取一个互斥锁(`pthread_mutex_lock`)以保证对全局变量`count`的操作是原子性的。线程分别将读取到的值加上10和20,然后更新`count`。
注意,由于`count`是`volatile`的,所以每次读写操作都是直接访问内存,避免了缓存带来的问题。然而,这个代码只是一个基础示例,实际应用中可能需要考虑更多的同步和错误处理细节。
相关推荐
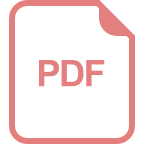
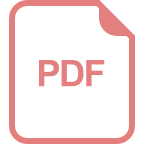
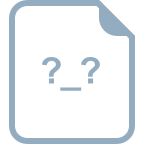














