三维装箱 python
时间: 2023-11-20 20:57:44 浏览: 66
三维装箱问题是一个NP-Hard问题,因此通常使用启发式算法来解决。以下是一些常用的解决方法:
1. 基于贪心算法的启发式方法:该方法将箱子按照体积从大到小排序,然后将它们依次放入箱子中,直到无法再放为止。这种方法的优点是简单易实现,但是不能保证得到最优解。
2. 基于遗传算法的启发式方法:该方法使用遗传算法来搜索最优解。首先生成一组随机解,然后使用遗传算法对这些解进行优化。这种方法的优点是可以得到较好的解,但是计算时间较长。
3. 基于模拟退火算法的启发式方法:该方法使用模拟退火算法来搜索最优解。首先生成一个随机解,然后使用模拟退火算法对这个解进行优化。这种方法的优点是可以得到较好的解,且计算时间较短。
以下是一个使用基于贪心算法的启发式方法来解决三维装箱问题的Python代码示例:
```python
class Box:
def __init__(self, id, l, w, h):
self.id = id
self.l = l
self.w = w
self.h = h
class Bin:
def __init__(self, l, w, h):
self.l = l
self.w = w
self.h = h
self.items = []
def add_item(self, item):
self.items.append(item)
def pack_boxes(boxes, bin):
boxes = sorted(boxes, key=lambda x: x.l * x.w * x.h, reverse=True)
for box in boxes:
if bin.l >= box.l and bin.w >= box.w and bin.h >= box.h:
bin.add_item(box)
bin.l -= box.l
bin.w -= box.w
bin.h -= box.h
boxes = [Box(1, 3, 4, 5), Box(2, 4, 5, 6), Box(3, 5, 6, 7)]
bin = Bin(10, 10, 10)
pack_boxes(boxes, bin)
for item in bin.items:
print(item.id)
```
相关推荐
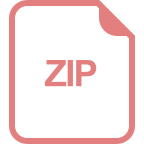














