C#窗体程序,运行程序时读取图片文件夹,图片文件夹里有10张图片,其中有4张图片上有特定标记,点击下一页按钮,根据图片的名称怎么判断出已经点到了有特定标记的图片的完整代码
时间: 2024-09-22 12:08:34 浏览: 36
在C#的Windows Forms应用程序中,你可以通过遍历文件夹、加载图片并检查图片内容的方式来实现这个功能。以下是一个简化的示例代码,假设你正在使用PictureBox控件显示图片,并有一个名为`IsMarked`的方法用于检查图片是否包含特定标记:
```csharp
using System;
using System.Drawing;
using System.IO;
public partial class MainForm : Form
{
private int currentPage = 0;
private string[] imageNames;
// 假设imageFolderPath是你存放图片的目录路径
private readonly string imageFolderPath = "path_to_your_images_folder";
public MainForm()
{
InitializeComponent();
LoadImages();
}
private void LoadImages()
{
imageNames = Directory.GetFiles(imageFolderPath, "*.jpg"); // 或者".png"等你实际使用的图片格式
pictureBox1.ImageLocation = GetImageFilePath(currentPage);
}
private string GetImageFilePath(int index)
{
if (index >= 0 && index < imageNames.Length)
return imageNames[index];
else
throw new IndexOutOfRangeException("Invalid page number");
}
private bool IsMarked(Image img)
{
// 这里只是一个示例,你需要替换为你的实际标记检测逻辑
Bitmap bitmap = new Bitmap(img);
// 检查图片是否有标记...
// 如果有标记,返回true;否则false
return CheckForMark(bitmap); // 假设CheckForMark是一个虚构的方法
}
private void nextPageButton_Click(object sender, EventArgs e)
{
currentPage++;
if (currentPage >= imageNames.Length)
MessageBox.Show("已到达最后一张图片。");
else
{
pictureBox1.ImageLocation = GetImageFilePath(currentPage);
if (IsMarked(pictureBox1.Image))
MessageBox.Show("当前图片包含特定标记!");
}
}
private bool CheckForMark(Bitmap bitmap) // 根据实际情况编写此方法
{
// 例如,如果标记是一个矩形区域,可以这样检查:
using (Graphics graphics = Graphics.FromImage(bitmap))
{
// ... 检测是否存在矩形区域...
return detectionResult; // 替换为真实的结果
}
}
}
```
阅读全文
相关推荐
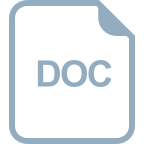
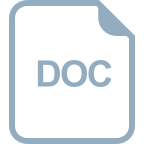
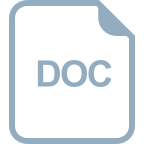

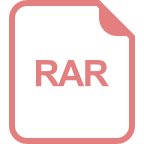
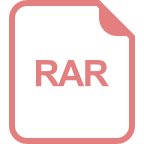
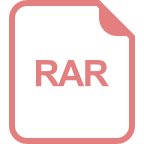
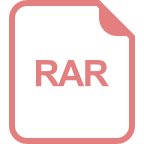
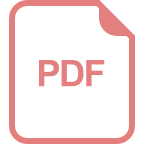
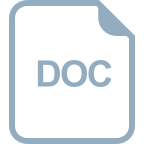
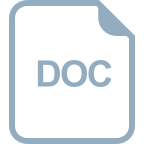
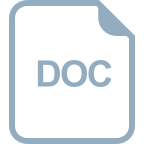
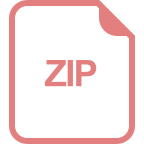
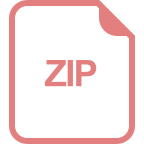
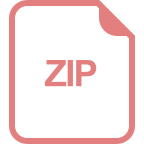
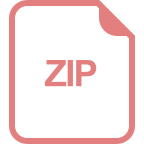