在C++中如何使用第三方库处理multipart/form-data?
时间: 2024-10-10 22:12:29 浏览: 145
在C++中处理`multipart/form-data`通常涉及到HTTP请求或文件上传,这时可以利用第三方库如C++版的`cpprestsdk`(来自Microsoft)或者`nlohmann/json`等来进行。以下是使用`cpprestsdk`处理multipart数据的基本步骤:
1. **安装依赖**:
首先,你需要从GitHub或其他官方渠道下载并安装cpprestsdk,比如通过`vcpkg`包管理器。
2. **包含头文件**:
包含必要的库头文件,如`websockets`、`http_client`以及`utility`中的`odata`模块。
```cpp
#include <cpprest/http_client.h>
#include <cpprest/filestream.h>
#include <cpprest/json.h>
```
3. **创建HTTP客户端**:
创建一个`http_client`实例,并设置需要的URL。
```cpp
http_client client(U("https://example.com/upload"));
```
4. **构建multipart body**:
使用`http_message`来构造multipart表单,添加部分(parts)包括键值对和文件流。
```cpp
http_message request(methods::POST);
request.set_request_uri("/upload");
request.headers().add(U("Content-Type"), U("multipart/form-data; boundary=myboundary"));
pplx::task<http_response> send_request = client.request(request);
```
5. **添加文件部分**:
使用`file_stream`从本地文件加载内容,然后添加到请求消息里。
```cpp
std::ifstream file("my_file.txt", std::ios::binary);
file_stream source(file);
source.content_type(U("text/plain")); // 设置文件类型
auto file_part = web::http::details::http_content(source, U("file_name.txt"), "myboundary");
request.add_body_part(file_part);
```
6. **发送请求并处理响应**:
执行请求任务并处理响应结果。
```cpp
try {
http_response response = await_send_request(send_request);
if (response.status_code() == status_codes::HTTP_OK) {
// 处理服务器返回的数据
} else {
throw std::runtime_error("Request failed with code " + to_string(response.status_code()));
}
} catch (const std::exception& e) {
// 错误处理
}
```
阅读全文
相关推荐


















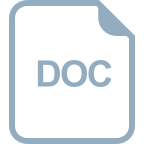