action = self.actor(state).cpu().data.numpy().flatten().reshape(1, -1)
时间: 2024-06-04 19:06:30 浏览: 111
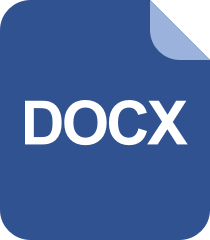
libgdx轻量级跨平台GUI库scene2d.ui教程

这段代码是一个深度强化学习中的 actor-critic 算法中,用于获取当前状态的行动概率分布的函数。其中,self.actor 是一个神经网络模型,输入为当前状态 state,输出为行动概率分布。通过调用该神经网络模型,获取当前状态下的行动概率分布,然后将其转换为一个一维数组(flatten)并重新 reshape 为一个 1 行 n 列的矩阵,其中 n 表示行动的数量。最后,使用 cpu() 和 data.numpy() 将结果转换为一个 numpy 数组。这个数组可以被用于产生随机行动、选择最优行动或进行其他后续处理。
阅读全文
相关推荐
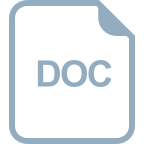
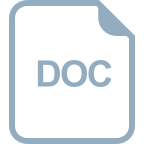

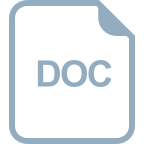
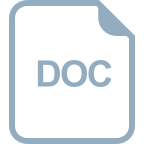