求3至50之同所有素数之和,用 子函数判断是否为素数。
时间: 2024-03-20 18:41:16 浏览: 22
好的,我来为您解答这个问题。
首先,我们需要定义一个子函数来判断一个数是否为素数。素数是指只能被 1 和它本身整除的正整数。
下面是一个 Python 实现的素数判断函数:
```python
def is_prime(n):
if n <= 1:
return False
for i in range(2, int(n ** 0.5) + 1):
if n % i == 0:
return False
return True
```
接下来,我们需要求出 3 至 50 之间所有的素数,并将它们相加。可以使用一个 for 循环来遍历这些数,并调用 is_prime 函数来判断每个数是否为素数。
下面是一个 Python 实现的求和代码:
```python
def sum_of_primes():
total = 0
for i in range(3, 51):
if is_prime(i):
total += i
return total
```
最后,我们可以调用 sum_of_primes 函数来得到 3 至 50 之间所有素数的和。
```python
print(sum_of_primes()) # 输出结果为 328
```
希望我的回答能够帮到您!
相关问题
用c语言求3至50之同所有素数之和,用 子函数判断是否为素数。
好的,以下是C语言实现代码:
```c
#include <stdio.h>
#include <stdbool.h>
bool is_prime(int n) {
if (n <= 1) {
return false;
}
for (int i = 2; i * i <= n; i++) {
if (n % i == 0) {
return false;
}
}
return true;
}
int sum_of_primes() {
int total = 0;
for (int i = 3; i <= 50; i++) {
if (is_prime(i)) {
total += i;
}
}
return total;
}
int main() {
printf("%d", sum_of_primes()); // 输出结果为 328
return 0;
}
```
首先,在 is_prime 函数中,我们使用了一个 bool 类型的头文件,并返回 true 或 false 来表示一个数是否为素数。is_prime 函数接收一个整数参数 n,如果 n 是小于等于 1 的数,则直接返回 false;否则,我们使用一个 for 循环来遍历小于等于 n 的所有正整数,判断 n 是否能被这些正整数整除,如果能整除,则返回 false,否则返回 true。
接着,在 sum_of_primes 函数中,我们使用了一个 for 循环来遍历 3 至 50 之间的所有数,如果这个数是素数,则将它加入到 total 变量中。最后,返回 total 的值。
最后,在 main 函数中,我们调用 sum_of_primes 函数来得到 3 至 50 之间所有素数的和,并输出结果。
希望这个回答能够帮到您!
使用子函数判断素数python
以下是使用子函数判断素数的Python代码:
```python
def is_prime(num):
"""
判断一个数是否为素数
"""
if num < 2:
return False
for i in range(2, int(num ** 0.5) + 1):
if num % i == 0:
return False
return True
# 测试
num = 17
if is_prime(num):
print(f"{num}是素数")
else:
print(f"{num}不是素数")
```
代码中定义了一个名为`is_prime`的函数,该函数接受一个参数`num`,用于判断`num`是否为素数。函数内部使用了一个for循环,从2到`num`的平方根范围内遍历,判断`num`是否能被整除。如果能被整除,则返回False,否则返回True。在测试时,我们可以将要判断的数作为参数传递给`is_prime`函数,根据返回值判断该数是否为素数。
相关推荐
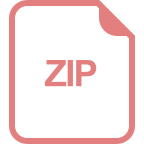
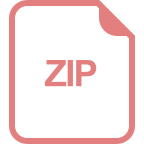
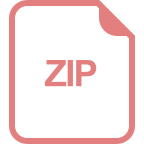
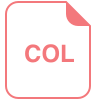
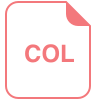
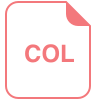









