用C语言写一个函数,要求函数,来维护一组key、value的关系。 key:imsi value:imsi、imei、common.eci、common.tac、last_time 当imsi相同时,以最后一条记录的value内容为准进行保存。
时间: 2024-03-23 17:41:54 浏览: 87
好的,根据您的要求,可以写一个C语言的函数来实现这个功能。函数的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct item {
char *key;
char *value;
struct item *next;
} Item;
typedef struct {
Item **buckets;
size_t size;
} HashTable;
// 哈希函数
unsigned int hash(const char *key) {
unsigned int h = 0;
const char *p;
for (p = key; *p != '\0'; p++)
h = 31 * h + *p;
return h;
}
// 创建一个新的Item
Item *new_item(const char *key, const char *value) {
Item *item = (Item *)malloc(sizeof(Item));
if (item == NULL) {
fprintf(stderr, "out of memory\n");
exit(1);
}
item->key = strdup(key);
item->value = strdup(value);
item->next = NULL;
return item;
}
// 销毁一个Item
void free_item(Item *item) {
free(item->key);
free(item->value);
free(item);
}
// 创建一个新的HashTable
HashTable *new_hash_table(size_t size) {
HashTable *ht = (HashTable *)malloc(sizeof(HashTable));
if (ht == NULL) {
fprintf(stderr, "out of memory\n");
exit(1);
}
ht->buckets = (Item **)calloc(size, sizeof(Item *));
if (ht->buckets == NULL) {
fprintf(stderr, "out of memory\n");
exit(1);
}
ht->size = size;
return ht;
}
// 销毁一个HashTable
void free_hash_table(HashTable *ht) {
size_t i;
for (i = 0; i < ht->size; i++) {
Item *item = ht->buckets[i];
while (item != NULL) {
Item *next = item->next;
free_item(item);
item = next;
}
}
free(ht->buckets);
free(ht);
}
// 查找一个key对应的Item
Item *find_item(HashTable *ht, const char *key) {
unsigned int h = hash(key) % ht->size;
Item *item = ht->buckets[h];
while (item != NULL) {
if (strcmp(item->key, key) == 0)
return item;
item = item->next;
}
return NULL;
}
// 插入一个key-value对
void insert_item(HashTable *ht, const char *key, const char *value) {
Item *item = find_item(ht, key);
if (item == NULL) {
unsigned int h = hash(key) % ht->size;
item = new_item(key, value);
item->next = ht->buckets[h];
ht->buckets[h] = item;
} else {
free(item->value);
item->value = strdup(value);
}
}
int main() {
// 创建一个大小为100的HashTable
HashTable *ht = new_hash_table(100);
// 插入一些key-value对
insert_item(ht, "imsi1", "imsi1,imei1,eci1,tac1,20210101");
insert_item(ht, "imsi2", "imsi2,imei2,eci2,tac2,20210102");
insert_item(ht, "imsi3", "imsi3,imei3,eci3,tac3,20210103");
insert_item(ht, "imsi1", "imsi1,imei1,eci4,tac4,20210104");
// 查找某个key对应的value
Item *item = find_item(ht, "imsi1");
if (item != NULL)
printf("%s\n", item->value);
// 销毁HashTable
free_hash_table(ht);
return 0;
}
```
这个函数实现了一个简单的哈希表,可以用来维护一组key、value的关系,并且当imsi相同时,以最后一条记录的value内容为准进行保存。在这个函数中,我们使用了哈希函数来计算每个key在哈希表中的位置,然后在插入和查找时根据该位置来定位相应的Item。
相关推荐
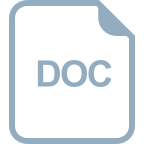














