写一个函数,来维护一组key、value的关系。 key:imsi value:imsi、imei、common.eci、common.tac、last_time 要求,imsi相同时,以最后一条记录的value内容为准进行保存。用C语言来写
时间: 2024-03-23 20:44:04 浏览: 43
下面是一个用C语言实现的维护key-value关系的函数,满足题目要求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STR_LEN 100
// 定义key-value对的结构体
typedef struct {
char *key;
char *value;
} KeyValue;
// 定义存储key-value对的结构体
typedef struct {
KeyValue *pairs;
int size;
int capacity;
} KeyValueStore;
// 初始化存储结构体
void initKeyValueStore(KeyValueStore *store) {
store->pairs = malloc(sizeof(KeyValue) * 10);
store->size = 0;
store->capacity = 10;
}
// 在存储结构体中查找指定key的value
char* lookupValue(KeyValueStore *store, char *key) {
for (int i = store->size - 1; i >= 0; i--) {
if (strcmp(store->pairs[i].key, key) == 0) {
return store->pairs[i].value;
}
}
return NULL;
}
// 在存储结构体中添加或更新指定key的value
void addOrUpdateValue(KeyValueStore *store, char *key, char *value) {
char *existingValue = lookupValue(store, key);
if (existingValue != NULL) {
free(existingValue);
} else if (store->size == store->capacity) {
store->capacity *= 2;
store->pairs = realloc(store->pairs, sizeof(KeyValue) * store->capacity);
}
store->pairs[store->size].key = strdup(key);
store->pairs[store->size].value = strdup(value);
store->size++;
}
// 释放存储结构体
void freeKeyValueStore(KeyValueStore *store) {
for (int i = 0; i < store->size; i++) {
free(store->pairs[i].key);
free(store->pairs[i].value);
}
free(store->pairs);
}
int main() {
KeyValueStore store;
initKeyValueStore(&store);
// 模拟添加key-value对
addOrUpdateValue(&store, "imsi1", "imsi1,imei1,eci1,tac1,100");
addOrUpdateValue(&store, "imsi2", "imsi2,imei2,eci2,tac2,200");
addOrUpdateValue(&store, "imsi1", "imsi1,imei3,eci3,tac3,300");
addOrUpdateValue(&store, "imsi3", "imsi3,imei4,eci4,tac4,400");
// 打印存储结构体中的key-value对
for (int i = 0; i < store.size; i++) {
printf("%s\n", store.pairs[i].value);
}
// 释放存储结构体
freeKeyValueStore(&store);
return 0;
}
```
这个程序中,我们使用了两个结构体:`KeyValue`用于存储单个key-value对,`KeyValueStore`用于存储多个key-value对。我们使用`initKeyValueStore()`函数初始化存储结构体,`lookupValue()`函数在存储结构体中查找指定key的value,`addOrUpdateValue()`函数在存储结构体中添加或更新指定key的value,`freeKeyValueStore()`函数释放存储结构体。在`main()`函数中,我们模拟添加了四个key-value对,并打印了存储结构体中的所有value。
阅读全文
相关推荐
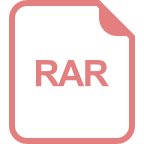
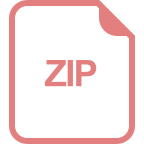










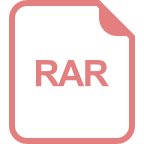
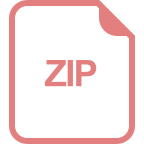
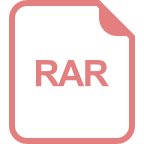
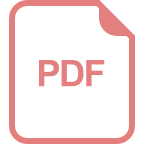
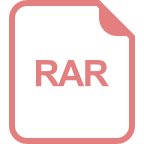
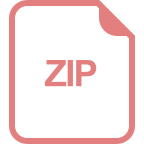