return data.drop_duplicates()
时间: 2024-05-22 18:16:36 浏览: 106
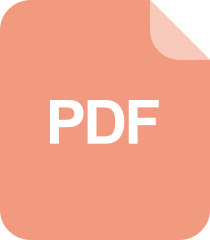
Python DataFrame使用drop_duplicates()函数去重(保留重复值,取重复值)

This code would remove all duplicated rows from the DataFrame 'data' and return the modified DataFrame. The 'drop_duplicates()' method checks for duplicated rows based on all columns by default, but you can specify specific columns by passing them as arguments to the method.
阅读全文
相关推荐
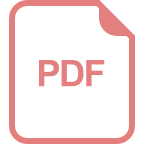
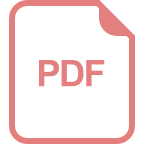












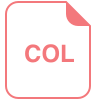
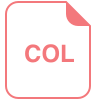

