python银行家算法实现资源分配和进程调度
时间: 2023-11-18 13:04:46 浏览: 49
很抱歉,Python并不是操作系统,也不是用于操作系统开发的编程语言,因此Python本身并没有实现银行家算法。但是,我们可以使用Python编写模拟银行家算法的程序来进行资源分配和进程调度的模拟。
以下是一个简单的Python程序,用于模拟银行家算法的资源分配和进程调度:
```python
定义银行家算法的数据结构
class Banker:
def __init__(self, available, max_need, allocation):
self.available = available # 可用资源
self.max_need = max_need # 进程最大需求量
self.allocation = allocation # 进程已分配资源量
self.need = max_need - allocation # 进程尚需资源量
# 检查是否存在安全序列
def is_safe(self):
work = self.available.copy()
finish = [False] * len(self.max_need)
safe_sequence = []
while True:
found = False
for i in range(len(self.max_need)):
if not finish[i] and all(need <= work for need, work in zip(self.need[i], work)):
work = [work[j] + self.allocation[i][j] for j in range(len(work))]
finish[i] = True
safe_sequence.append(i)
found = True
if not found:
break
return all(finish), safe_sequence
# 分配资源
def allocate(self, process_id, request):
if all(request <= self.need[process_id]) and all(request <= self.available):
self.available -= request
self.allocation[process_id] += request
self.need[process_id] -= request
return True
else:
return False
# 释放资源
def release(self, process_id, release):
self.available += release
self.allocation[process_id] -= release
self.need[process_id] += release
# 测试程序
if __name__ == '__main__':
# 初始化银行家算法的数据结构
available = [3, 3, 2]
max_need = [[7, 5, 3], [3, 2, 2], [9, 0, 2], [2, 2, 2], [4, 3, 3]]
allocation = [[0, 1, 0], [2, 0, 0], [3, 0, 2], [2, 1, 1], [0, 0, 2]]
banker = Banker(available, max_need, allocation)
# 模拟进程请求资源
process_id = 0
request = [0, 2, 0]
if banker.allocate(process_id, request):
print('分配成功')
if banker.is_safe()[0]:
print('存在安全序列')
else:
print('不存在安全序列,分配失败')
banker.release(process_id, request)
else:
print('分配失败')
```
相关推荐
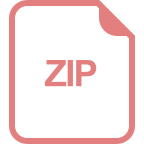
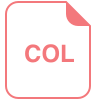
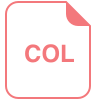
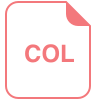








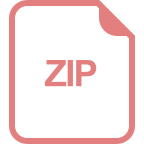