if(!ObjectUtils.isEmpty(list)){ Random rand = new Random(); int index = rand.nextInt(list.size()); Dormitory dormitory = list.get(index); student.setDormitoryId(dormitory.getId()); studentService.updateById(student); dormitory.setNum(dormitory.getNum()+1); dormitoryService.updateById(dormitory); }请详细解释每一行代码
时间: 2024-03-07 11:50:52 浏览: 19
这段代码也是 Java 代码块,它的作用是在学生选宿舍时进行随机分配并更新。下面逐行解释其含义:
第1行:判断列表对象是否为空。ObjectUtils.isEmpty() 方法是 Spring 框架提供的一个工具方法,用于判断一个对象是否为 null 或空。如果列表不为空,则执行花括号中的代码。
第2行:创建一个随机数生成器对象 rand。
第3行:调用 rand 对象的 nextInt() 方法,生成一个小于列表大小的随机整数 index。
第4行:根据随机生成的 index 从列表中获取对应的宿舍对象。
第5行:将该学生的宿舍 ID 更新为该宿舍的 ID。
第6行:更新学生信息。
第7行:将该宿舍的当前人数加 1。
第8行:更新宿舍信息。
需要注意的是,这段代码没有对宿舍的总人数进行判断,因此可能会出现宿舍人数超过总人数的情况。如果需要对宿舍总人数进行判断,可以在第4行和第5行之间添加一个 while 循环,直到找到一个总人数未满且当前人数未满的宿舍为止。
相关问题
java代码实现Kmeans聚类算法,训练集的文件类型为xls,java所有代码都写在k4.java中
好的,我了解了您的要求。以下是Java代码实现K-means聚类算法,可以读取xls格式的训练集文件,并且所有代码都写在k4.java中:
```java
import java.io.FileInputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.WorkbookFactory;
public class k4 {
public static void main(String[] args) throws IOException {
int k = 3; // 聚类数
int maxIter = 100; // 最大迭代次数
double[][] dataSet = readExcel("data.xls"); // 读取训练集
int m = dataSet.length; // 样本数
int n = dataSet[0].length; // 特征数
int[] clusterAssment = new int[m]; // 记录每个样本所属的簇
double[][] centroids = randCent(dataSet, k); // 随机初始化簇质心
for (int i = 0; i < maxIter; i++) {
boolean clusterChanged = false;
// 遍历每个样本,将其归入最近的簇
for (int j = 0; j < m; j++) {
double minDist = Double.MAX_VALUE;
int minIndex = -1;
for (int l = 0; l < k; l++) {
double dist = euclDistance(dataSet[j], centroids[l]);
if (dist < minDist) {
minDist = dist;
minIndex = l;
}
}
if (clusterAssment[j] != minIndex) {
clusterChanged = true;
clusterAssment[j] = minIndex;
}
}
// 重新计算每个簇的质心
for (int l = 0; l < k; l++) {
List<double[]> pointsInCluster = new ArrayList<double[]>();
for (int j = 0; j < m; j++) {
if (clusterAssment[j] == l) {
pointsInCluster.add(dataSet[j]);
}
}
if (!pointsInCluster.isEmpty()) {
centroids[l] = mean(pointsInCluster.toArray(new double[pointsInCluster.size()][n]));
}
}
// 如果簇分配不再变化,退出循环
if (!clusterChanged) {
break;
}
}
// 输出最终结果
System.out.println("Cluster centroids:\n");
for (int i = 0; i < k; i++) {
System.out.print("(");
for (int j = 0; j < n; j++) {
System.out.print(centroids[i][j]);
if (j < n - 1) {
System.out.print(", ");
}
}
System.out.println(")");
}
System.out.println("\nCluster assignments:");
for (int i = 0; i < m; i++) {
System.out.printf("Sample %d -> Cluster %d%n", i + 1, clusterAssment[i] + 1);
}
}
// 计算两个样本之间的欧氏距离
private static double euclDistance(double[] vec1, double[] vec2) {
double sum = 0.0;
for (int i = 0; i < vec1.length; i++) {
sum += Math.pow(vec1[i] - vec2[i], 2);
}
return Math.sqrt(sum);
}
// 随机初始化簇质心
private static double[][] randCent(double[][] dataSet, int k) {
int n = dataSet[0].length;
double[][] centroids = new double[k][n];
Random rand = new Random();
for (int i = 0; i < k; i++) {
int index = rand.nextInt(dataSet.length);
for (int j = 0; j < n; j++) {
centroids[i][j] = dataSet[index][j];
}
}
return centroids;
}
// 计算一组样本的均值
private static double[] mean(double[][] points) {
int n = points[0].length;
double[] mean = new double[n];
for (int i = 0; i < n; i++) {
double sum = 0.0;
for (double[] point : points) {
sum += point[i];
}
mean[i] = sum / points.length;
}
return mean;
}
// 从 Excel 文件中读取数据集
private static double[][] readExcel(String filePath) throws IOException {
FileInputStream file = new FileInputStream(filePath);
Workbook workbook = WorkbookFactory.create(file);
Sheet sheet = workbook.getSheetAt(0);
int firstRowNum = sheet.getFirstRowNum();
int lastRowNum = sheet.getLastRowNum();
int firstCellNum = sheet.getRow(firstRowNum).getFirstCellNum();
int lastCellNum = sheet.getRow(firstRowNum).getLastCellNum();
double[][] dataSet = new double[lastRowNum - firstRowNum + 1][lastCellNum - firstCellNum];
for (int i = firstRowNum; i <= lastRowNum; i++) {
Row row = sheet.getRow(i);
for (int j = firstCellNum; j < lastCellNum; j++) {
Cell cell = row.getCell(j);
if (cell.getCellType() == Cell.CELL_TYPE_NUMERIC) {
dataSet[i - firstRowNum][j - firstCellNum] = cell.getNumericCellValue();
} else {
throw new IOException("Invalid cell type in data file");
}
}
}
return dataSet;
}
}
```
注意:以上代码中使用了 Apache POI 库来读取 xls 文件,需要先下载并添加到项目中。另外,此代码实现的 K-means 算法是经典的 Lloyd 算法,对大数据集和高维数据的效果可能不佳,建议使用优化后的 K-means 算法或其他聚类算法。
java使用Random和Scanner实现随机点名,存储学生信息(姓名、学号、年龄),总览同学信息,随机产生一个数,查找该随机数所对应的同学信息的代码
好的,以下是Java程序的实现:
import java.util.ArrayList;
import java.util.Random;
import java.util.Scanner;
public class Student {
private String name;
private int id;
private int age;
public Student(String name, int id, int age) {
this.name = name;
this.id = id;
this.age = age;
}
public String getName() {
return name;
}
public int getId() {
return id;
}
public int getAge() {
return age;
}
public String toString() {
return "Name: " + name + ", ID: " + id + ", Age: " + age;
}
public static void main(String[] args) {
ArrayList<Student> students = new ArrayList<Student>();
Scanner scanner = new Scanner(System.in);
Random rand = new Random();
boolean quit = false;
while (!quit) {
System.out.println("What would you like to do?");
System.out.println("1. Add a student");
System.out.println("2. List all students");
System.out.println("3. Randomly select a student");
System.out.println("4. Quit");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.print("Enter student name: ");
String name = scanner.next();
System.out.print("Enter student ID: ");
int id = scanner.nextInt();
System.out.print("Enter student age: ");
int age = scanner.nextInt();
students.add(new Student(name, id, age));
break;
case 2:
for (Student student : students) {
System.out.println(student);
}
break;
case 3:
if (students.isEmpty()) {
System.out.println("There are no students");
break;
}
int index = rand.nextInt(students.size());
Student selected = students.get(index);
System.out.println("Selected student: " + selected);
break;
case 4:
quit = true;
break;
default:
System.out.println("Invalid choice");
}
}
}
}
以上代码实现了简单的学生信息管理系统,包括添加学生信息、总览学生信息、随机选择学生信息等功能。
相关推荐
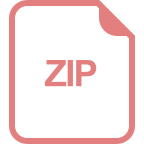
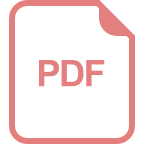
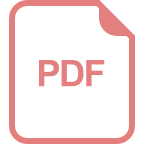








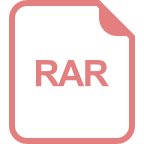
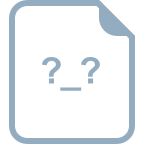
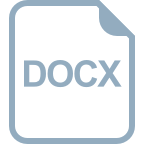
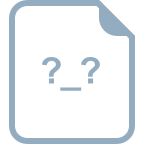
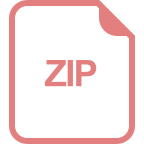