java ftp上传文件
时间: 2023-06-30 12:04:30 浏览: 50
要使用Java上传文件到FTP服务器,您需要使用FTPClient类。以下是一个简单的示例:
```java
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import java.io.FileInputStream;
import java.io.IOException;
public class FTPUploader {
public static void main(String[] args) {
String server = "ftp.example.com";
int port = 21;
String user = "username";
String password = "password";
String localFilePath = "/path/to/local/file.txt";
String remoteFilePath = "/path/to/remote/file.txt";
FTPClient ftpClient = new FTPClient();
FileInputStream fis = null;
try {
ftpClient.connect(server, port);
ftpClient.login(user, password);
ftpClient.enterLocalPassiveMode();
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
fis = new FileInputStream(localFilePath);
boolean success = ftpClient.storeFile(remoteFilePath, fis);
if (success) {
System.out.println("File uploaded successfully.");
} else {
System.out.println("File upload failed.");
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fis != null) {
fis.close();
}
ftpClient.logout();
ftpClient.disconnect();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
在上面的示例中,我们使用了Apache Commons Net库中的FTPClient类。我们首先连接到FTP服务器,并使用用户名和密码进行身份验证。然后,我们输入被动模式,并设置文件类型为二进制。接下来,我们打开要上传的本地文件,使用storeFile()方法将其上传到FTP服务器上指定的远程路径中。最后,我们关闭文件输入流,注销FTP客户端并断开连接。
请注意,您需要将Apache Commons Net库添加到类路径中,以便使用FTPClient类。
阅读全文
相关推荐
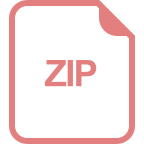
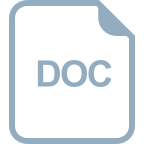



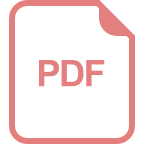
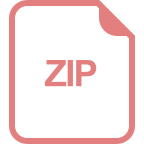
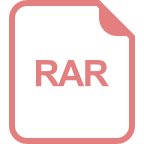




