springboot 线程池并行化处理
时间: 2023-07-06 13:36:30 浏览: 45
在Spring Boot中,可以使用Java线程池来实现并行化处理。具体步骤如下:
1. 在Spring Boot的配置文件中配置线程池相关参数,例如线程池大小等。
2. 在需要并行化处理的方法上添加@Async注解,表示该方法将在另一个线程中异步执行。
3. 在调用需要并行化处理的方法时,使用Spring Boot的异步调用方式,例如CompletableFuture。
4. 在异步调用方法后,可以使用CompletableFuture的一些方法等待异步方法执行完毕,并获取结果。
示例代码如下:
```java
// 1. 在配置文件中配置线程池
@Configuration
@EnableAsync
public class AppConfig implements AsyncConfigurer {
@Override
public Executor getAsyncExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(10);
executor.setMaxPoolSize(100);
executor.setQueueCapacity(10);
executor.initialize();
return executor;
}
}
// 2. 在需要并行化处理的方法上添加@Async注解
@Service
public class MyService {
@Async
public CompletableFuture<String> doSomethingAsync() {
// 需要异步执行的方法
return CompletableFuture.completedFuture("done");
}
}
// 3. 在调用需要并行化处理的方法时,使用异步调用方式
@RestController
public class MyController {
@Autowired
private MyService myService;
@GetMapping("/async")
public CompletableFuture<String> async() {
return myService.doSomethingAsync();
}
}
// 4. 在异步调用方法后,可以使用CompletableFuture的一些方法等待异步方法执行完毕,并获取结果
CompletableFuture<String> future = myService.doSomethingAsync();
String result = future.get();
```
这样就可以使用线程池实现Spring Boot的并行化处理了。
相关推荐
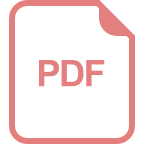














